Dynamic email templates
Create email templates that populate in real-time with dynamic data unique to each recipient. With variables and dynamic content, you can turn every transactional email into a personalized touchpoint for your user experience.

Build once, personalize infinitely
Work more efficiently by creating dynamic email templates once and deploying personalized messages at scale. Minimize repetitive tasks and keep your system clean and free from excessive templates and workflows.
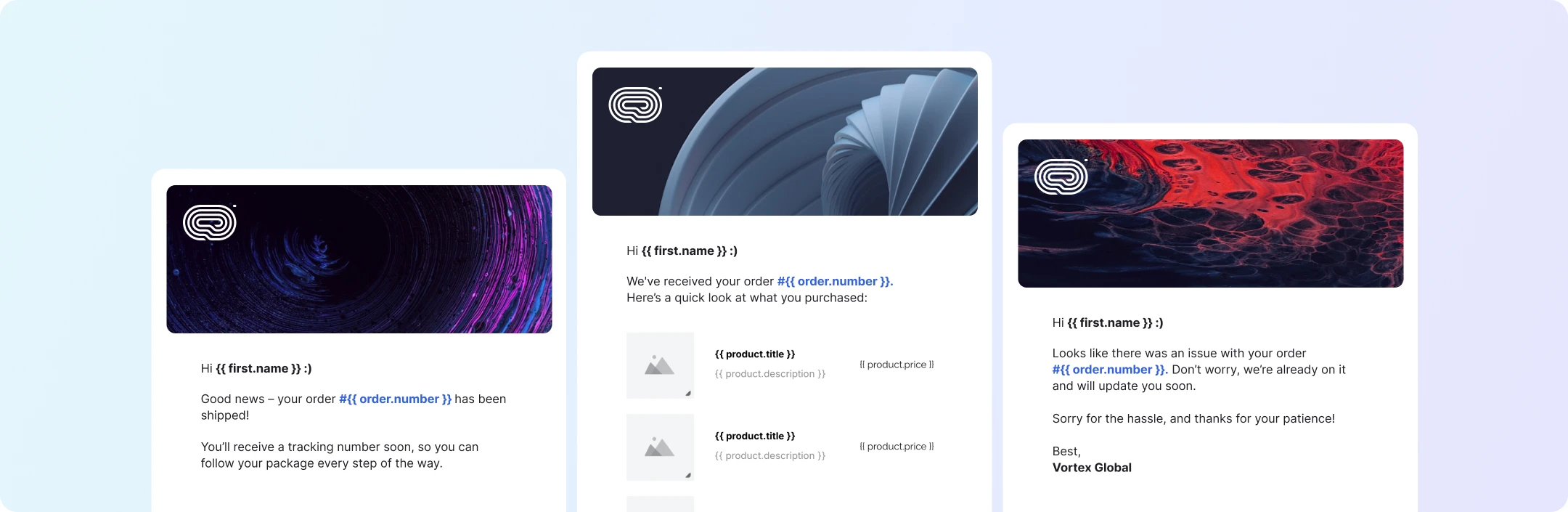
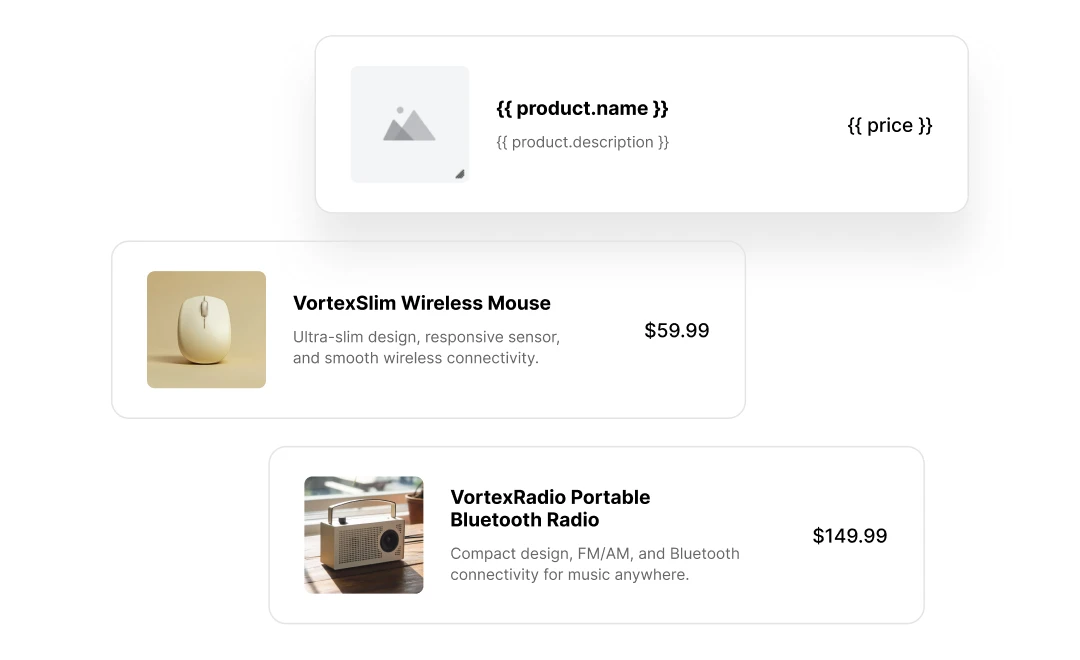
Deliver tailored experiences with personalized content
Enhance the user experience by using variables, filters, conditional logic, and dynamic tables in your templates. Dynamically generate order information, account activity, product recommendations, and more.
Template creation made easy with 3 powerful builders
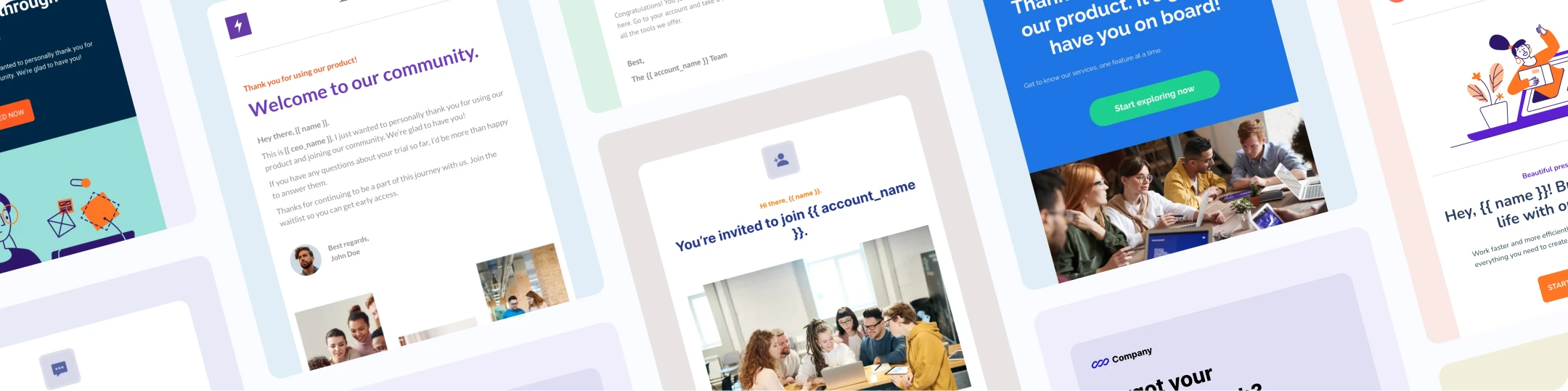
Drag & drop: No coding needed
Design professional and responsive dynamic templates with 90+ prebuilt blocks, including dynamic tables and products.
HTML: Build your own
Write from scratch or import existing HTML templates. Save time with snippets, automatic CSS formatting, error tagging, and more.
Rich-text: Simple formatting power
Create professional plain-text emails with simple inline editing and added HTML formatting in a few clicks.
Send dynamic email templates quickly with our API
curl -X POST \
https://api.mailersend.com/v1/email \
-H 'Content-Type: application/json' \
-H 'X-Requested-With: XMLHttpRequest' \
-H 'Authorization: Bearer {place your token here without brackets}' \
-d '{
"from": {
"email": "your@email.com"
},
"to": [
{
"email": "your@client.com"
}
],
"subject": "Hello from MailerSend!",
"text": "Greetings from the team, you got this message through MailerSend.",
"html": "Greetings from the team, you got this message through MailerSend.
"
}'
import { MailerSend, EmailParams, Sender, Recipient } from "mailersend";
const mailerSend = new MailerSend({
api_key: "key",
});
const sentFrom = new Sender("you@yourdomain.com", "Your name");
const recipients = [
new Recipient("your@client.com", "Your Client")
];
const emailParams = new EmailParams()
.setFrom(sentFrom)
.setTo(recipients)
.setReplyTo(sentFrom)
.setSubject("This is a Subject")
.setHtml("This is the HTML content")
.setText("This is the text content");
await mailerSend.email.send(emailParams);
$mailersend = new MailerSend();
$recipients = [
new Recipient('your@client.com', 'Your Client'),
];
$emailParams = (new EmailParams())
->setFrom('your@email.com')
->setFromName('Your Name')
->setRecipients($recipients)
->setSubject('Subject')
->setHtml('Greetings from the team, you got this message through MailerSend.')
->setText('Greetings from the team, you got this message through MailerSend.');
$mailersend->email->send($emailParams);
php artisan make:mail ExampleEmail
Mail::to('you@client.com')->send(new ExampleEmail());
from mailersend import emails
mailer = emails.NewEmail()
mail_body = {}
mail_from = {
"name": "Your Name",
"email": "your@domain.com",
}
recipients = [
{
"name": "Your Client",
"email": "your@client.com",
}
]
mailer.set_mail_from(mail_from, mail_body)
mailer.set_mail_to(recipients, mail_body)
mailer.set_subject("Hello!", mail_body)
mailer.set_html_content("Greetings from the team, you got this message through MailerSend.
", mail_body)
mailer.set_plaintext_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.send(mail_body)
require "mailersend-ruby"
# Intialize the email class
ms_email = Mailersend::Email.new
# Add parameters
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_from("email" => "your@domain.com", "name" => "Your Name")
ms_email.add_subject("Hello!")
ms_email.add_text("Greetings from the team, you got this message through MailerSend.")
ms_email.add_html("Greetings from the team, you got this message through MailerSend.")
# Send the email
ms_email.send
package main
import (
"context"
"os"
"fmt"
"time"
"github.com/mailersend/mailersend-go"
)
func main() {
// Create an instance of the mailersend client
ms := mailersend.NewMailersend(os.Getenv("MAILERSEND_API_KEY"))
ctx := context.Background()
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
subject := "Subject"
text := "This is the text content"
html := "This is the HTML content
"
from := mailersend.From{
Name: "Your Name",
Email: "your@domain.com",
}
recipients := []mailersend.Recipient{
{
Name: "Your Client",
Email: "your@client.com",
},
}
// Send in 5 minute
sendAt := time.Now().Add(time.Minute * 5).Unix()
tags := []string{"foo", "bar"}
message := ms.Email.NewMessage()
message.SetFrom(from)
message.SetRecipients(recipients)
message.SetSubject(subject)
message.SetHTML(html)
message.SetText(text)
message.SetTags(tags)
message.SetSendAt(sendAt)
message.SetInReplyTo("client-id")
res, _ := ms.Email.Send(ctx, message)
fmt.Printf(res.Header.Get("X-Message-Id"))
}
import com.mailersend.sdk.Email;
import com.mailersend.sdk.MailerSend;
import com.mailersend.sdk.MailerSendResponse;
import com.mailersend.sdk.exceptions.MailerSendException;
public void sendEmail() {
Email email = new Email();
email.setFrom("name", "your email");
email.addRecipient("name", "your@recipient.com");
// you can also add multiple recipients by calling addRecipient again
email.addRecipient("name 2", "your@recipient2.com");
// there's also a recipient object you can use
Recipient recipient = new Recipient("name", "your@recipient3.com");
email.AddRecipient(recipient);
email.setSubject("Email subject");
email.setPlain("This is the text content");
email.setHtml("This is the HTML content
");
MailerSend ms = new MailerSend();
ms.setToken("Your API token");
try {
MailerSendResponse response = ms.emails().send(email);
System.out.println(response.messageId);
} catch (MailerSendException e) {
e.printStackTrace();
}
}
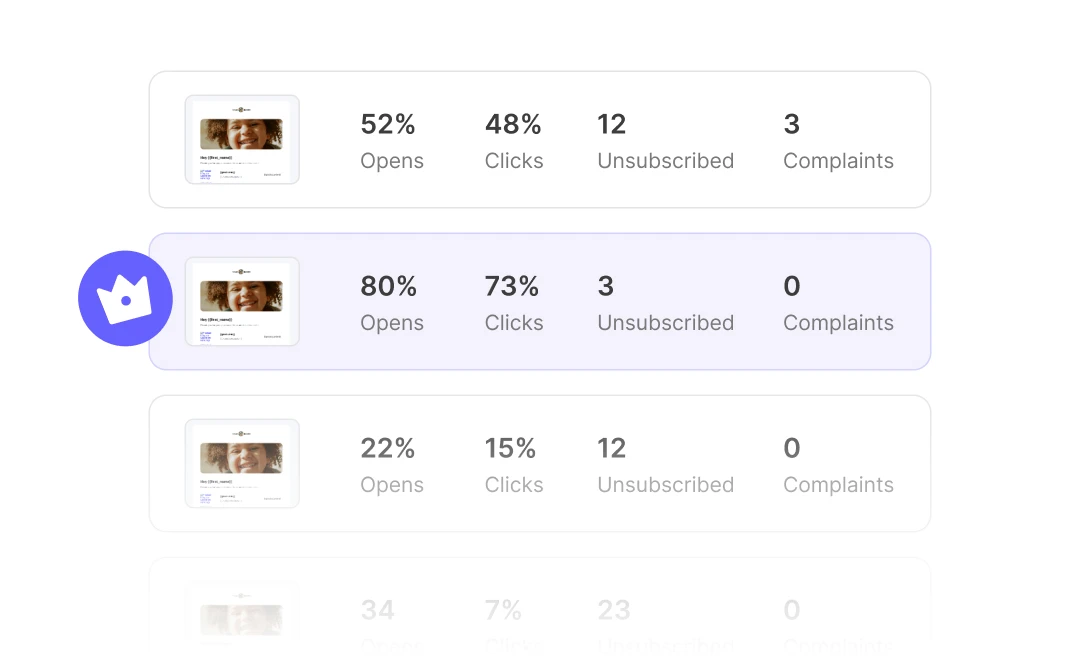
Optimize templates with data-driven insights
Create up to 5 versions of a template and use email split testing to find which performs the best. Analyze the insights to improve your designs, boost engagement, and increase conversions.
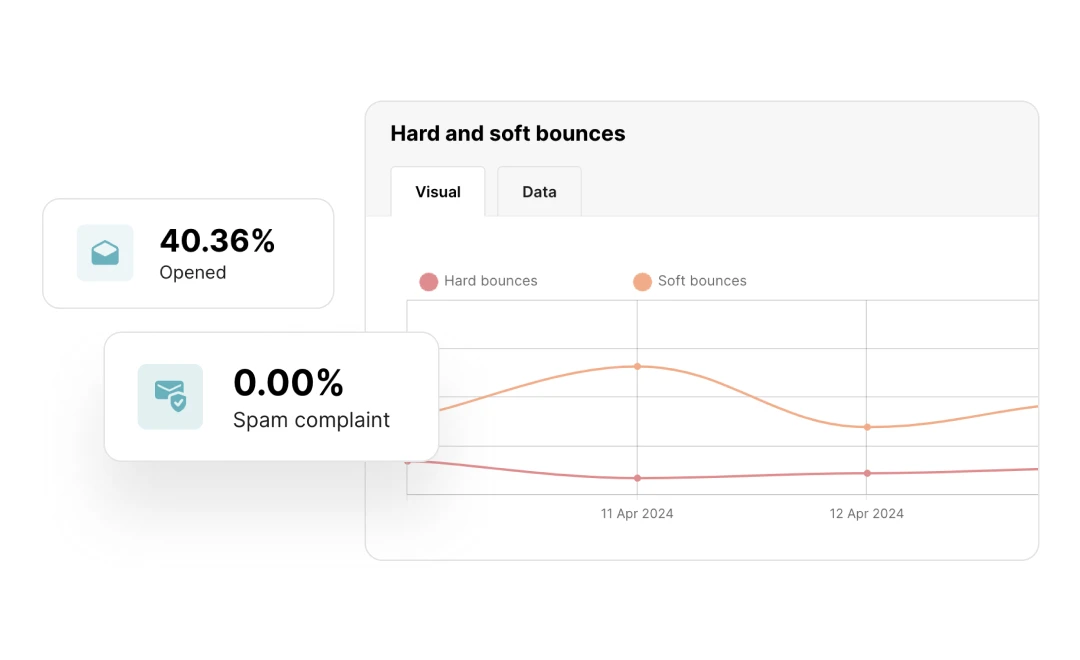
Monitor performance with in-depth analytics
Access key metrics such as opens, clicks, bounces, unsubscribes, and more in the analytics dashboard. Filter data for your account, domains, or individual email templates and create custom reports.
Email delivery you can count on
Fast, reliable delivery
Built for the maximum deliverability of high volumes of email, MailerSend helps you to reach more inboxes and avoid spam folders.
Simple, robust email API
Our dev-friendly email API is simple to implement and advanced in functionality. No/low-code options and integrations available.
Support of the human-kind
Starting out or leveling up: get help when you need it most from our friendly, award-winning, customer support team.
What our customers have to say
Frequently Asked Questions
What is a dynamic email template?
A dynamic email template is a type of email that includes variables to personalize the content for each individual recipient. A simple example of this is using the {{name}} variable to add the recipient’s name.
What is a dynamic email used for?
In transactional emails, dynamic templates are used to send emails with account or transaction-specific content (such as account details, password reset link or order confirmation) to recipients. Dynamic email email content can also be used to personalize messaging in order to build relationships and increase engagement, for example, by translating strings of text and adjusting it to the recipient’s locale.
How can I create dynamic email?
You can create a dynamic email by adding personalization variables into your API call. Learn how to use variables.
More features to explore
Send emails
Email delivery
Enjoy the flexibility of sending a few emails or scaling quickly to send a few million.
Transactional emails
Intuitively-designed tools allow anyone to contribute, while an advanced infrastructure lets you scale fast.
SMTP relay
Use Simple Mail Transfer Protocol when you want to quickly send emails using a reliable Internet standard.
Email API
Start sending and tracking your emails with our easy API integration process and clean documentation.
Email verification
Verify a single email address or upload an entire email list to verify in bulk.
Email address validation API
Keep your recipient list clean and maintain great deliverability by automatically verifying incoming email addresses with the email address validation API.
Control your sendings
Webhooks
Get notified as email events happen so your integration can automatically trigger reactions.
Advanced email tracking
Every email is a learning experience. Monitor your email performance to find what works best.
Manage the unsubscribe page
Whether people are unsubscribing, give them a compelling reason to stay.
Email suppression list management
Protect your sending reputation by adding email addresses and domains that you should not send to.
Activity and performance logs
Easy access to detailed API and SMTP activity data and template error logs.
Track the results
Create emails
Custom HTML email builder
Interested in writing your own HTML code? Our HTML email template editor gives you the flexibility to build exactly what you want.
Drag & drop email template builder
Our drag & drop email editor empowers you to create professionally-designed transactional emails.
Rich-text email editor
Create plain text emails with the formatting capabilities of HTML. Add links, images, bullet points and style text with ease.
Email split testing
Email split testing will improve engagement with your transactional emails by helping you learn what your customers want more.
Send surveys
Understand your customers and users on a deeper level and gain valuable insights to help you improve your product and customer experience with surveys.
Control your account
User management
Invite your team members to collaborate on projects by assigning roles and granting permissions.
File manager
Streamline your workflow by organizing and keeping all your files in one place in the cloud.
Multiple domains
Use multiple domains to manage different brands or products with one MailerSend account.
Dedicated IP
Take control of your sending reputation with your own dedicated IP address and optimize your sendings for improved deliverability.
MailerSend iOS app
Access email activity, domain settings, and analytics on the go with MailerSend iOS app.
Start collaborating on transactional emails now
Try MailerSend for free: Design your templates and send up to 100 emails with a trial domain. Add your own domain to get 3,000 emails/month free and start sending.