Email address validation API
Keep your recipient list clean and maintain great deliverability by automatically verifying incoming email addresses with the email address validation API.

Validate email addresses in real time
curl -X POST \
https://api.mailersend.com/v1/email \
-H 'Content-Type: application/json' \
-H 'X-Requested-With: XMLHttpRequest' \
-H 'Authorization: Bearer {place your token here without brackets}' \
-d '{
"from": {
"email": "your@email.com"
},
"to": [
{
"email": "your@client.com"
}
],
"subject": "Hello from MailerSend!",
"text": "Greetings from the team, you got this message through MailerSend.",
"html": "Greetings from the team, you got this message through MailerSend."
}'
use MailerSend\MailerSend;
use MailerSend\Helpers\Builder\Recipient;
use MailerSend\Helpers\Builder\EmailParams;
$mailersend = new MailerSend(['api_key' => 'key']);
$recipients = [
new Recipient('your@client.com', 'Your Client'),
];
$emailParams = (new EmailParams())
->setFrom('your@email.com')
->setFromName('Your Name')
->setRecipients($recipients)
->setSubject('Subject')
->setHtml('Greetings from the team, you got this message through MailerSend.')
->setText('Greetings from the team, you got this message through MailerSend.');
$mailersend->email->send($emailParams);
const Recipient = require("mailersend").Recipient;
const EmailParams = require("mailersend").EmailParams;
const MailerSend = require("mailersend");
const mailersend = new MailerSend({
api_key: "key",
});
const recipients = [new Recipient("your@client.com", "Your Client")];
const emailParams = new EmailParams()
.setFrom("your@email.com")
.setFromName("Your Name")
.setRecipients(recipients)
.setSubject("Subject")
.setHtml("Greetings from the team, you got this message through MailerSend.")
.setText("Greetings from the team, you got this message through MailerSend.");
mailersend.send(emailParams);
package main
import (
"context"
"fmt"
"time"
"github.com/mailersend/mailersend-go"
)
var APIKey string = "Api Key Here"
func main() {
ms := mailersend.NewMailersend(APIKey)
ctx := context.Background()
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
subject := "Subject"
text := "Greetings from the team, you got this message through MailerSend."
html := "Greetings from the team, you got this message through MailerSend."
from := mailersend.From{
Name: "Your Name",
Email: "your@email.com",
}
recipients := []mailersend.Recipient{
{
Name: "Your Client",
Email: "your@client.com",
},
}
variables := []mailersend.Variables{
{
Email: "your@client.com",
Substitutions: []mailersend.Substitution{
{
Var: "foo",
Value: "bar",
},
},
},
}
tags := []string{"foo", "bar"}
message := ms.NewMessage()
message.SetFrom(from)
message.SetRecipients(recipients)
message.SetSubject(subject)
message.SetHTML(html)
message.SetText(text)
message.SetSubstitutions(variables)
message.SetTags(tags)
res, _ := ms.Send(ctx, message)
fmt.Printf(res.Header.Get("X-Message-Id"))
}
from mailersend import emails
mailer = emails.NewEmail()
mail_body = {}
mail_from = {
"name": "Your Name",
"email": "your@domain.com",
}
recipients = [
{
"name": "Your Client",
"email": "your@client.com",
}
]
mailer.set_mail_from(mail_from, mail_body)
mailer.set_mail_to(recipients, mail_body)
mailer.set_subject("Hello!", mail_body)
mailer.set_html_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.set_plaintext_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.send(mail_body)
require "mailersend-ruby"
# Intialize the email class
ms_email = Mailersend::Email.new
# Add parameters
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_from("email" => "your@domain.com", "name" => "Your Name")
ms_email.add_subject("Hello!")
ms_email.add_text("Greetings from the team, you got this message through MailerSend.")
ms_email.add_html("Greetings from the team, you got this message through MailerSend.")
# Send the email
ms_email.send
Check an entire list with bulk email verification
Post a GET request to verify an entire list of email addresses via the API. Ensure your database is always clean and up-to-date and protect your sender reputation.
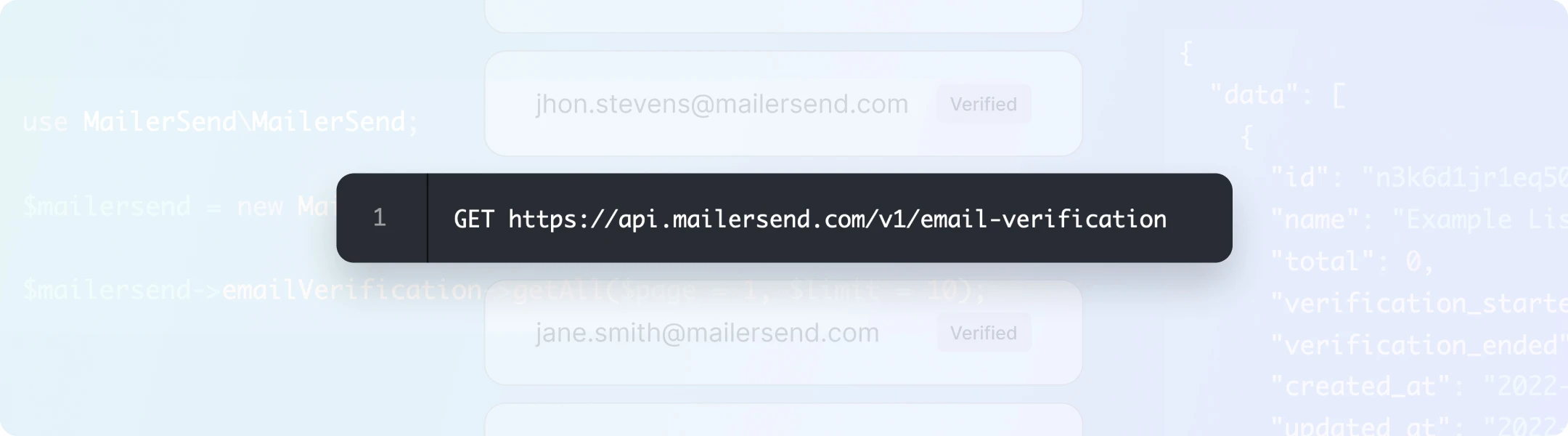
Fast, reliable email verification API
Comprehensive results
Detect catch-all and role-based emails, full mailboxes, syntax errors, typos, disposable email addresses, and blocked mailboxes.
Maximize deliverability
Improve your deliverability by filtering out invalid emails before they have a chance to damage your sender reputation.
Lower bounce rate
Reduce the number of invalid emails that reach your list without having to lift a finger and see your hard bounce rate decrease.
Reduce sending costs
Ensure only real customers are added to your list and send to people who will open and engage with your emails.
Improve the user experience
Let users know when they’ve entered an incorrect email address so they can easily fix the issue and continue on the customer journey.
Collect all potential leads
Maximize your sales potential and avoid losing leads because they’ve entered the wrong email address.
Real-time email verification use cases
-
E-commerce
Improve the user experience on account creation or checkout. Ensure customers receive all vital communication about their purchases such as shipping notifications and invoices. -
SaaS
Validate emails when new users sign up or change their account email address for a smooth user experience. Be sure that users can receive important notifications or password resets. -
Banks & fintech
Check customers’ emails when they update their contact information so they can avoid losing access to their account and continue to receive important communication.
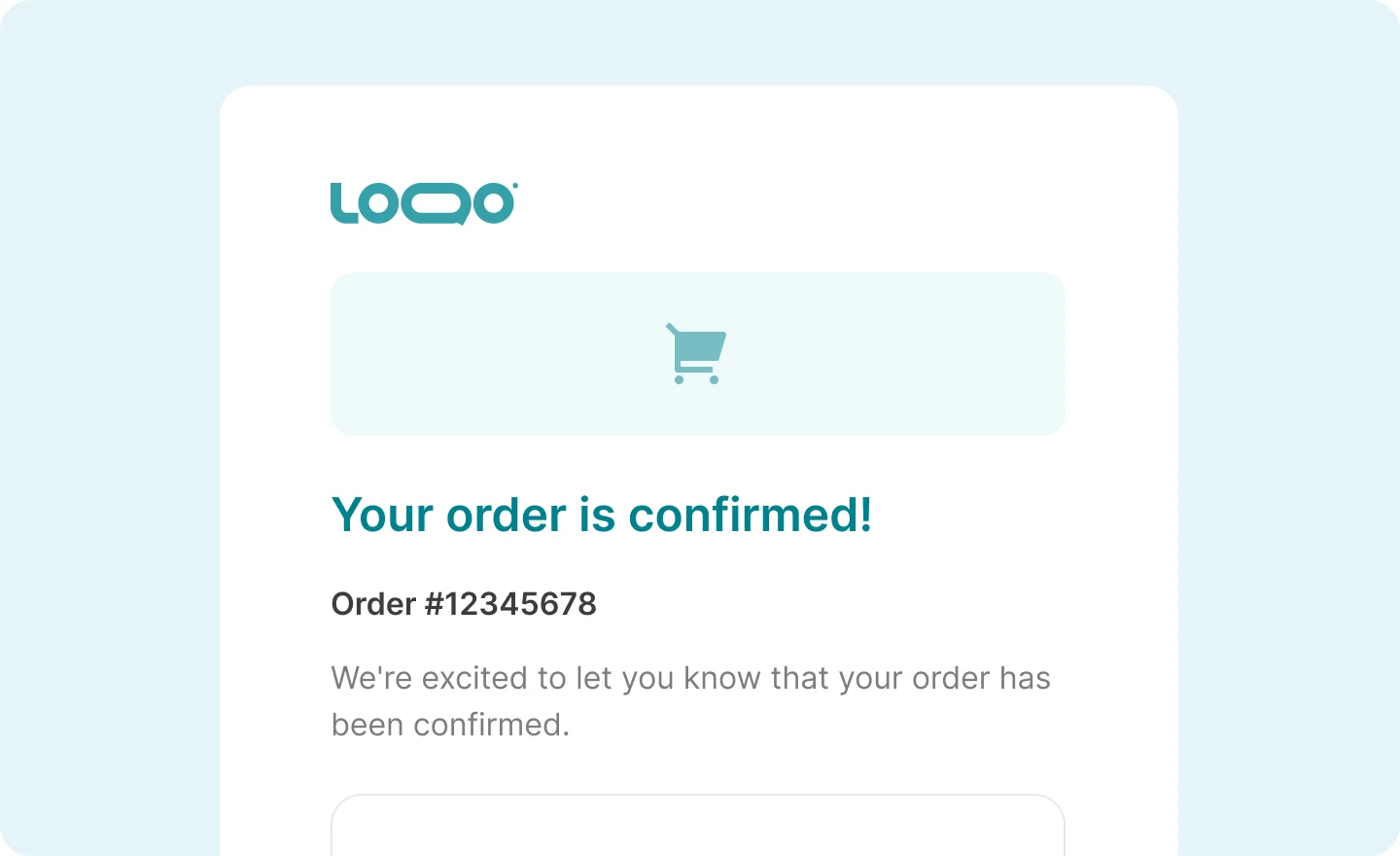
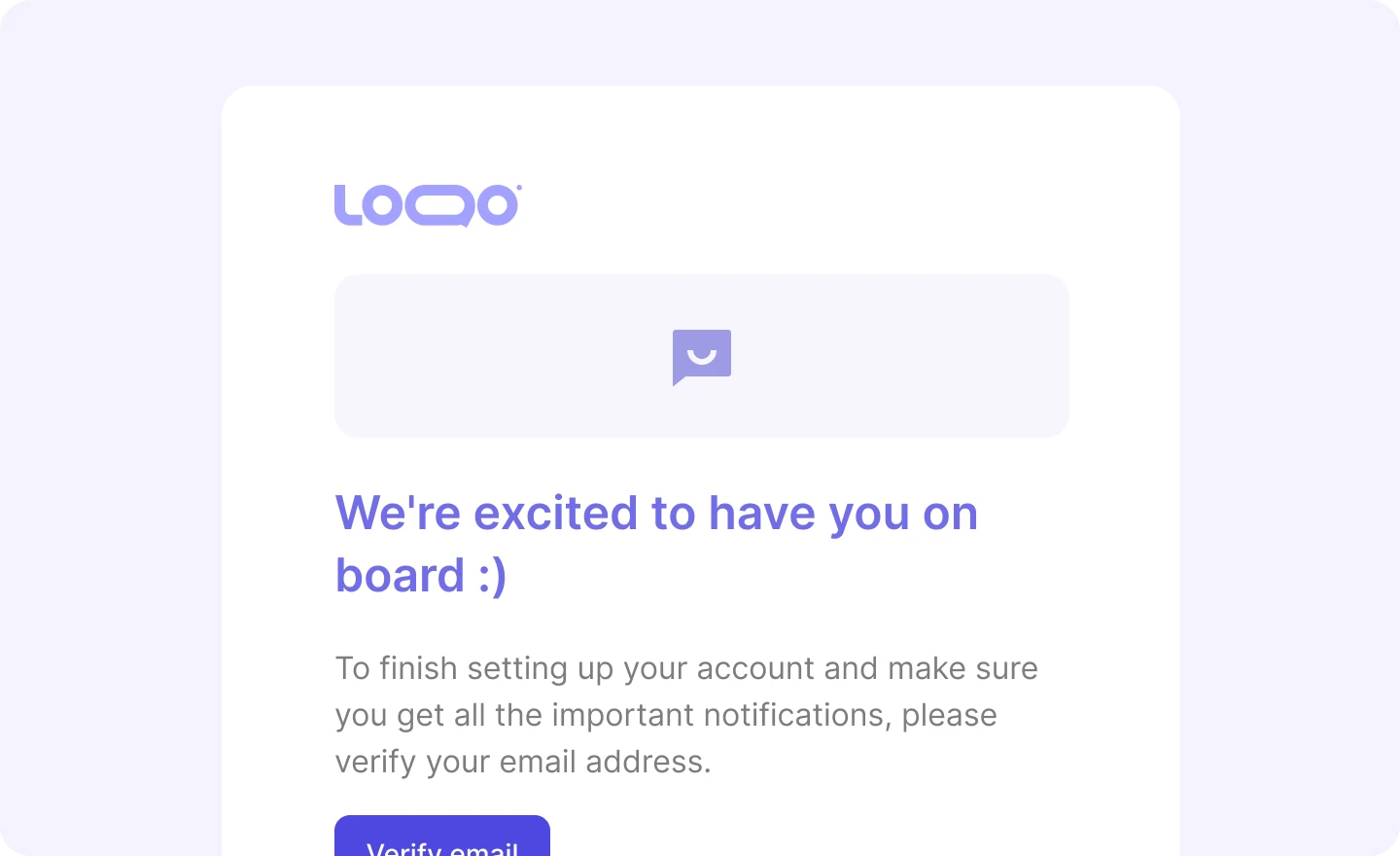
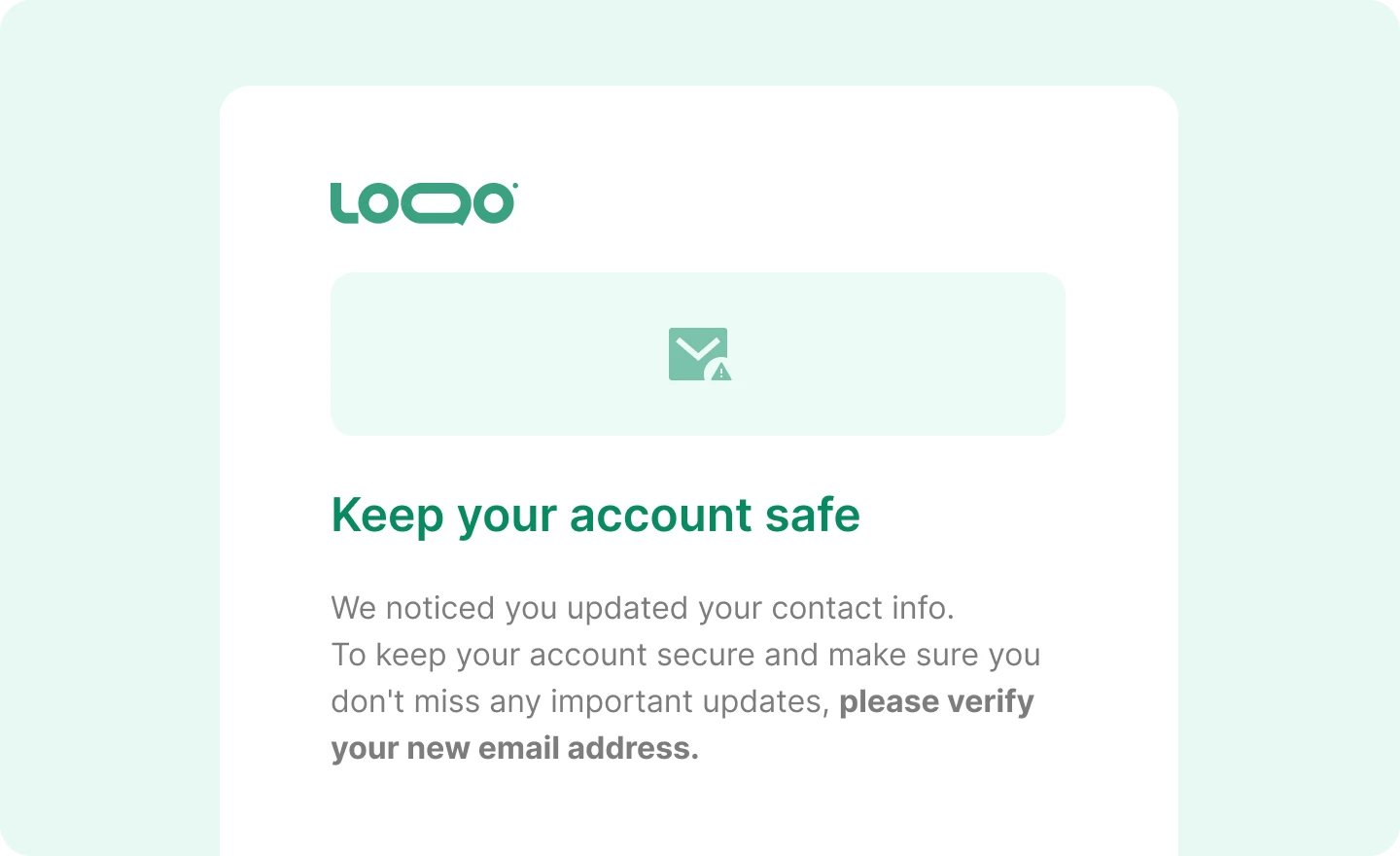
Integrations
MailerSend pricing
Hobby
Starter
Professional
Enterprise
Improve your transactional email delivery today
Get started with 3,000 emails/month free and access to MailerSend’s email API, templates, and more. Test it out with a trial domain—no credit card needed.
Frequently asked questions
How does real-time email validation work?
Real-time email validation via API works by integrating an email validation service into your application using an API key. When an email address is entered, the API performs a series of checks on your behalf via API calls, automating the verification process. The check includes syntax validation, domain verification, MX record lookup, and sometimes SMTP verification to confirm the email's validity and deliverability. It returns the results immediately.
How does email validation impact email deliverability and sender reputation?
Email validation allows you to identify and remove invalid email addresses that can harm your sender reputation and deliverability. By sending to a clean email list with real recipients, your deliverability and sender reputation will be improved and protected from the negative impacts of sending to bad email addresses.
Can the email address validation API be integrated with any email platform?
MailerSend’s email verifier is for use with the MailerSend email delivery platform.
Do I need a paid plan to use the email validation API endpoint?
You don’t need a paid plan but you do need to purchase email verification credits for the validation to be run successfully. Paid accounts will receive a number of free email address verification credits.