Sign up for a free account
A more intuitive Resend alternative for developers
Enjoy simple email sending with advanced features that you can quickly integrate into your app or website without the need for complex configuration.
Built for developers, designed for teams
Get started right now with our powerful email API
curl -X POST \
https://api.mailersend.com/v1/email \
-H 'Content-Type: application/json' \
-H 'X-Requested-With: XMLHttpRequest' \
-H 'Authorization: Bearer {place your token here without brackets}' \
-d '{
"from": {
"email": "your@email.com"
},
"to": [
{
"email": "your@client.com"
}
],
"subject": "Hello from MailerSend!",
"text": "Greetings from the team, you got this message through MailerSend.",
"html": "Greetings from the team, you got this message through MailerSend.
"
}'
use MailerSend\MailerSend;
use MailerSend\Helpers\Builder\Recipient;
use MailerSend\Helpers\Builder\EmailParams;
$mailersend = new MailerSend(['api_key' => 'key']);
$recipients = [
new Recipient('your@client.com', 'Your Client'),
];
$emailParams = (new EmailParams())
->setFrom('your@email.com')
->setFromName('Your Name')
->setRecipients($recipients)
->setSubject('Subject')
->setHtml('Greetings from the team, you got this message through MailerSend.
')
->setText('Greetings from the team, you got this message through MailerSend.');
$mailersend->email->send($emailParams);
php artisan make:mail ExampleEmail
Mail::to('you@client.com')->send(new ExampleEmail());
const Recipient = require("mailersend").Recipient;
const EmailParams = require("mailersend").EmailParams;
const MailerSend = require("mailersend");
const mailersend = new MailerSend({
api_key: "key",
});
const recipients = [new Recipient("your@client.com", "Your Client")];
const emailParams = new EmailParams()
.setFrom("your@email.com")
.setFromName("Your Name")
.setRecipients(recipients)
.setSubject("Subject")
.setHtml("Greetings from the team, you got this message through MailerSend.
")
.setText("Greetings from the team, you got this message through MailerSend.");
mailersend.send(emailParams);
package main
import (
"context"
"fmt"
"time"
"github.com/mailersend/mailersend-go"
)
var APIKey string = "Api Key Here"
func main() {
ms := mailersend.NewMailersend(APIKey)
ctx := context.Background()
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
subject := "Subject"
text := "Greetings from the team, you got this message through MailerSend."
html := "Greetings from the team, you got this message through MailerSend.
"
from := mailersend.From{
Name: "Your Name",
Email: "your@email.com",
}
recipients := []mailersend.Recipient{
{
Name: "Your Client",
Email: "your@client.com",
},
}
variables := []mailersend.Variables{
{
Email: "your@client.com",
Substitutions: []mailersend.Substitution{
{
Var: "foo",
Value: "bar",
},
},
},
}
tags := []string{"foo", "bar"}
message := ms.NewMessage()
message.SetFrom(from)
message.SetRecipients(recipients)
message.SetSubject(subject)
message.SetHTML(html)
message.SetText(text)
message.SetSubstitutions(variables)
message.SetTags(tags)
res, _ := ms.Send(ctx, message)
fmt.Printf(res.Header.Get("X-Message-Id"))
}
from mailersend import emails
mailer = emails.NewEmail()
mail_body = {}
mail_from = {
"name": "Your Name",
"email": "your@domain.com",
}
recipients = [
{
"name": "Your Client",
"email": "your@client.com",
}
]
mailer.set_mail_from(mail_from, mail_body)
mailer.set_mail_to(recipients, mail_body)
mailer.set_subject("Hello!", mail_body)
mailer.set_html_content("Greetings from the team, you got this message through MailerSend.
", mail_body)
mailer.set_plaintext_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.send(mail_body)
require "mailersend-ruby"
# Intialize the email class
ms_email = Mailersend::Email.new
# Add parameters
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_from("email" => "your@domain.com", "name" => "Your Name")
ms_email.add_subject("Hello!")
ms_email.add_text("Greetings from the team, you got this message through MailerSend.")
ms_email.add_html("Greetings from the team, you got this message through MailerSend.")
# Send the email
ms_email.send
Create simple SMTP integrations
Get up to 50 SMTP users on a Starter plan or unlimited on Professional. Then simply enter your SMTP credentials into your app, website, or plugin to start sending.
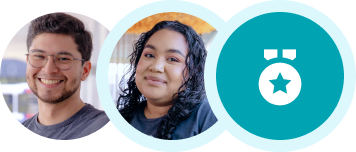
Fast and friendly support 24/7
Whether you’re just starting out or a well-seasoned sender, our award-winning customer support team is on hand and ready to assist.
Reliable suppressions handling
Avoid sending to hard-bounced emails, spam complaints, and unsubscribes. Harmful emails are automatically added to your suppression list the moment they are rejected.
Automatic domain verification
Seamlessly add DKIM, SPF, and RETURN-PATH authentication records to your DNS without leaving the MailerSend app, via a secure connection with Entri.
Detailed activity logs
Easily troubleshoot sending issues with full visibility into sending activity and errors. Get in-depth information about each email sent including error codes, endpoints, user agents, and more.
Comprehensive documentation from start to finish
We’ve got guides to help you every step of the way. Access extensive API documentation and technical guides, plus knowledge base articles that explain every feature in the app.
Template creation made easy in 3 ways
Drag & drop email builder
Leave email template creation to your design team thanks to the user-friendly drag-and-drop interface and pre-built blocks.
HTML email editor
Easily upload pre-built HTML templates or code from scratch. Optimize template creation with snippets, syntax highlights, and error tagging.
Rich-text
Create plain text templates with added HTML formatting for simple but professional-looking emails.
Straightforward webhook configuration
Benefit from real-time event monitoring by using webhooks to trigger activity notifications. Easily create new webhooks in the app or via API.
Inbound message processing
Use inbound routing to effortlessly manage incoming emails. MailerSend parses the message and forwards it to a specific email address or endpoint for integration with your app or CRM.
In-depth reporting and insights
Intuitive dashboard
Instantly get an overview of the most important metrics for your account so you can easily monitor overall health and performance.
Custom analytics reports
Get analytics for email volume, bounces, engagement, location, and more. Download and save reports for future use at the click of a button.
Real-time activity
Monitor deliverability with real-time activity statuses for each email. See when emails are queued, sent, delivered, bounced, and more.
Dedicated IPs
Dedicated IP addresses are available for high-volume senders with an Enterprise account, who prefer to take full control of their sender reputation and deliverability.
Transactional SMS API
Integrate high-volume, toll-free text messages with our SMS API and send OTPs, 2FA texts, reminders, and more. Currently available in North America.
Pricing
Hobby
Starter
Professional
Enterprise
Send transactional emails in 5 minutes
Send an email from the trial domain
Try out the features & check your activity
How MailerSend features compare with Resend
|
|
---|
|
|
|
---|---|---|
Email API, SMTP relay | ||
Webhooks | 50 | 10 |
SMS API | ||
Email Marketing SSO | ||
Inbound routing | ||
Suppression list management | ||
Email tracking & analytics | ||
Email split testing | ||
Drag & drop template builder | ||
HTML template editor | ||
GDPR compliance | ||
2FA | ||
API permissions | ||
IP allowlist | ||
Premium IP pool management | ||
Dedicated IP address | Available for high-volume senders | Available for high-volume senders |
Deliverability consultation | Available for Professional and Enterprise | |
iOS app | ||
Email verification | ||
Slack integration | ||
24/7 email support | Limited email/ticket support available | |
Live chat support | ||
Multiple users | 5 | 5 |
User roles | 5 roles and custom options are available | |
Multiple domains | 10 | 10 |
Integrations
FAQs
What is Resend?
Resend started out as an open source project, and has since become an email service provider made for developers to integrate transactional and marketing emails into their app or website.
Can I easily migrate from Resend to MailerSend?
We’ve made it super easy to get started with MailerSend; simply sign up for a free account and test out our capabilities with a trial domain. You can then subscribe to a free or paid plan, verify your domain, and use our API and integrations to implement MailerSend into your system.
What are the main differences between MailerSend and Resend?
While both solutions are built for developers, MailerSend offers a more user-friendly and intuitive interface and features to allow other non-technical team members to contribute to tasks such as template creation. MailerSend also offers a transactional SMS API, pre-built email templates, inbound routing, email verification, and other features.
Is MailerSend suitable for high-volume sending?
Yes, MailerSend’s infrastructure is built to handle high volumes of emails.
Switch to MailerSend today
Sign up free—no credit card needed—and start testing immediately with a free trial domain. Like what you see? Subscribe to a free or paid plan, verify your domain, and start sending!