How to validate email addresses with PHP: 3 methods explained
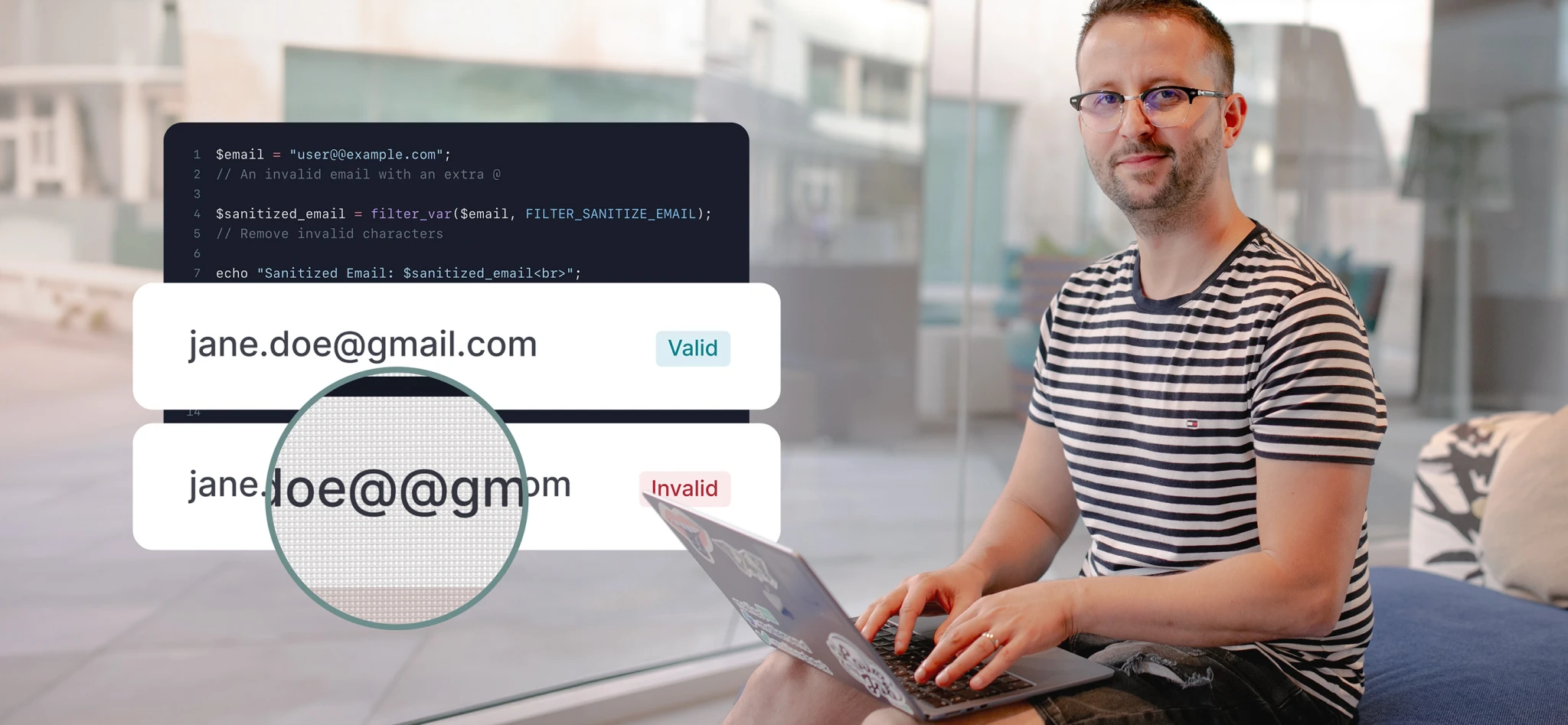
- PHP validation methods
- PHP email validation with filter_var()
- Handling invalid email addresses
- PHP email validation with regex
- Testing and debugging regex
- PHP email verification with an API
- How to set up PHP email verification with an API
- Combining an API with regex
- Validating emails with PHP in bulk
- Email validation matters… a lot
- Skipping email validation? Think twice
At best, not using email address validation will result in your database being cluttered with invalid email addresses and your customers having a less-than-stellar experience. At worst, it will harm your sender reputation and deliverability, and even present security risks and abuse of your platform. Yikes.
But there is a solution. Email validation prevents invalid email addresses from making it past your signup page, either by filtering out emails with invalid characters and syntax issues or with deeper verification checks.
In this guide, we’ll cover why email validation is really important, the 3 main PHP validation methods: PHP functions, regex, and APIs, and everything else you need to know to get started with validating emails using PHP. Let’s dive in.
Methods of validating emails with PHP
There are three ways to validate email addresses with PHP, and the one you choose depends on your use case and how accurate you need the validation to be.
The native PHP function filter_var() is a simple way to detect whether an email address follows PHP standards in regards to syntax and allowed characters. FILTER_VALIDATE_EMAIL checks the email format, while FILTER_SANITIZE_EMAIL sanitizes an invalid email address to remove unauthorized characters such as spaces, commas and other special characters.
The second method uses regular expressions or regex to create custom rules about the email addresses you allow to be entered. This is a bit more complex, as you’ll need to write and maintain your regex, but it allows you to use patterns or define specific criteria, such as domains.
Finally, for more advanced validation, a third-party API is the best method. Rather than simple email validation, an API performs an email verification check, meaning it can check for syntax errors as well as other types of invalid emails, including typos, full mailboxes, and disposable emails.
Feature | Native PHP Functions | Regex (preg_match()) | Third-Party API (e.g., MailerSend) |
Ease of Use | Very easy | More complex, requires writing & maintaining regex | Easy, but requires API setup & integration |
Accuracy | Checks for proper email format and allowed characters | Can be overly strict or too permissive | Best accuracy (verifies domain, DNS MX record lookup, etc.) |
Detects Typos (@gmial.com instead of @gmail.com) | No | No | Yes (Some APIs offer typo correction) |
Checks Domain Existence | No | No | Yes |
Verifies MX (Mail Exchange) Records | No | No | Yes |
Detects Disposable/Temporary Emails | No | No, but can be manually configured to filter them out | Yes (API can block temp email services) |
Prevents Fake/Spam Emails | No | No | Yes (API can flag known spam emails) |
Best Use Case | Simple email format validation | When you need a custom validation rule | When accuracy is critical (e.g., signups, transactions) |
PHP email validation with native functions ( filter_var() )
The built-in filter_var() function is used to filter and validate all kinds of data. Combine it with the FILTER_VALIDATE_EMAIL and FILTER_SANITIZE_EMAIL filters, and you have a simple and effective way to validate emails in your apps, databases or forms without the need for regex.
FILTER_VALIDATE_EMAIL checks that email addresses comply with PHP standards, so it only checks that an email follows the correct format:
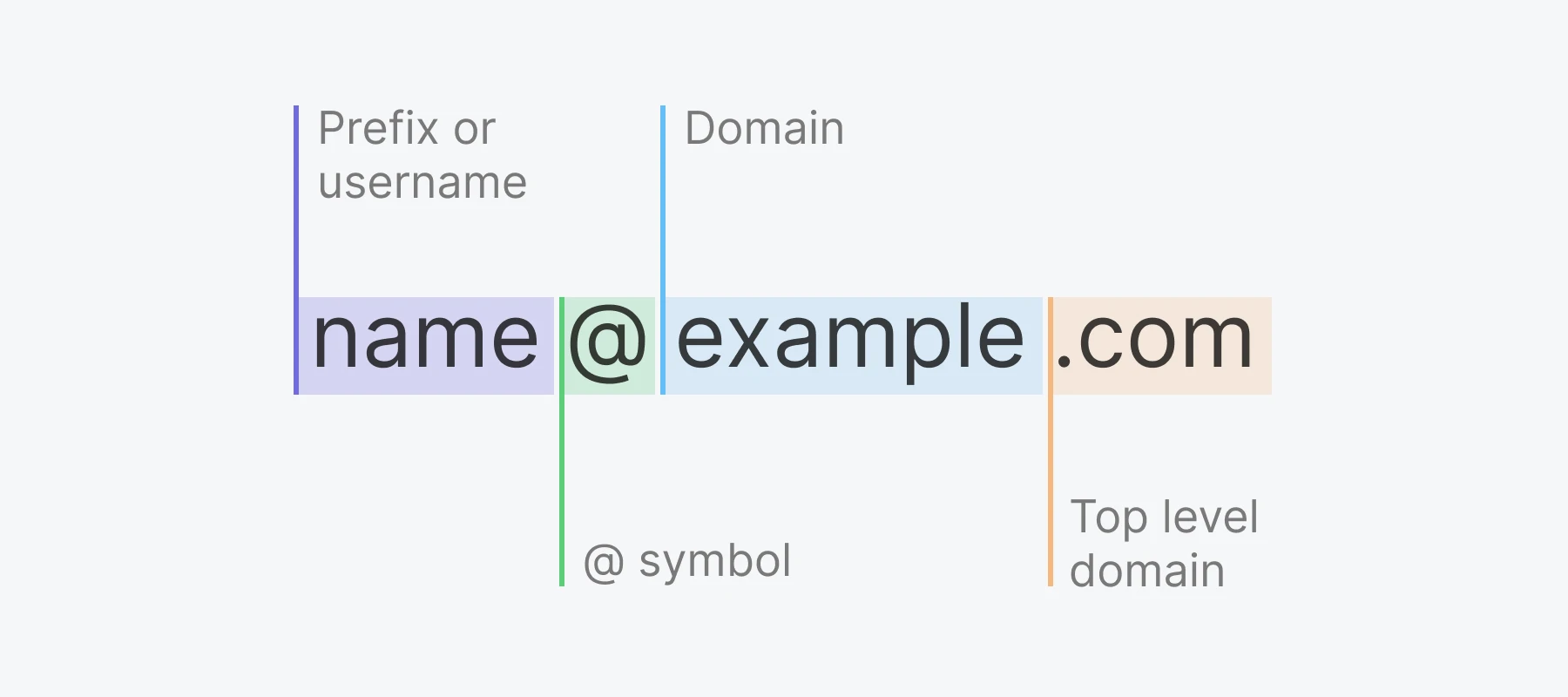
The function checks that the email contains a prefix or username, a domain part and top-level domain (TLD), and the '@' symbol connecting the two.
FILTER_SANITIZE_EMAIL checks for and removes non-permitted characters from the email address. This includes more than one ‘@’ symbol, spaces, commas, and other special characters excluding ‘ ~!$%^&*_=+}{'?- ’.
This is especially useful for removing potentially harmful characters that could be used for XSS and SQL injection attacks.
The PHP filter_var() function has limited support for international email addresses that use special characters (like the Greek alphabet, for example) and some newer TLDs. This means the function will return email addresses that use them as invalid.
So, let’s take a look at an example:
$email = "user@example.com";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Valid email address.";
} else {
echo "Invalid email address.";
}
In this script, if $email is a valid email according to PHP standards, filter_var($email, FILTER_VALIDATE_EMAIL) will return the email address itself. If $email is invalid, the function will return false.
Here’s another example using the FILTER_SANITIZE_EMAIL filter:
$email = "user#@example.com"; // An email with an invalid character
$sanitized_email = filter_var($email, FILTER_SANITIZE_EMAIL); // Remove invalid characters
echo "Sanitized Email: $sanitized_email
";
if (filter_var($sanitized_email, FILTER_VALIDATE_EMAIL)) {
echo "Valid email address.";
} else {
echo "Invalid email address.";
}
This time, filter_var($email, FILTER_SANITIZE_EMAIL) strips the invalid, ‘#’ symbol and returns the sanitized email. The sanitized email is then validated with FILTER_VALIDATE_EMAIL.
Handling invalid email addresses
To improve the user experience, your form should give feedback about invalid emails so that the user can correct it. Here’s an example of the code for a form and the PHP script for returning an error message.
<?php
// Initialize an empty error message
$error_message = "";
// Check if the form is submitted
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// Get and trim the email input to remove unnecessary spaces
$email = trim($_POST["email"]);
// Validate the email format
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$error_message =
"The email address you have entered is invalid. Please try again.";
} else {
// If the email is valid, you can process it further (e.g., save to a database)
// No success message is displayed
}
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Signup Form</title>
<style>
.error { color: red; font-weight: bold; }
</style>
</head>
<body>
<h2>Signup Form</h2>
<form action="<?php echo htmlspecialchars(
$_SERVER["PHP_SELF"]
); ?>" method="post">
<label for="email">Email:</label>
<input type="text" name="email" id="email"
value="<?php echo isset($email)
? htmlspecialchars($email)
: ""; ?>">
<input type="submit" value="Sign Up">
</form>
<?php if ($error_message): ?>
<p class="error"><?php echo $error_message; ?></p>
<?php endif; ?>
</body>
</html>
It’s a good idea to keep a log of all email validations so you can monitor for suspicious activity such as bot attacks. It can also help you with debugging if your script behaves in an unexpected way.
To include custom validation rules when using the built-in PHP functions, you can combine it with regular expressions. Let’s take a look at validating email addresses with regex.
Validating email addresses with regular expressions (regex)
PHP functions allow for basic email address validation based on standard criteria. Regex, on the other hand, allows you to validate emails with custom specifications outside of PHP’s built-in capabilities. For example, you could enforce a minimum or maximum length of a domain name, block specific domain names (particularly handy for filtering out temporary emails), or allow newer TLDs.
This makes the regex method a more effective solution if you need to handle international email addresses, have a high number of disposable domains signing up, or need to create any kind of custom validation rule.
Here’s a simple email validation code example using regex:
function isValidEmail($email) {
// Define a simple regex pattern for email validation
$pattern = '/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/';
// Use preg_match() to check if the email matches the pattern
if (preg_match($pattern, $email)) {
return "Valid email.";
} else {
return "Invalid email format.";
}
}
The regex pattern: $pattern = '/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/' includes the sequence of characters that defines the pattern in a valid email address. If the email matches the pattern: if (preg_match($pattern, $email)), it returns a valid status: { return "Valid email."; }. If not, the email is returned as invalid: else { return "Invalid email format." }.
Here’s an example of a more customized regex that blocks certain temporary email domains:
function isValidEmail($email) {
// Define a regex pattern for email validation and blocking certain domains
$pattern = '/^[a-zA-Z0-9._%+-]+@(?!tempmail\.com$|guerrillamail\.com$|10minutemail\.com$)[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/';
// Use preg_match() to check if the email matches the pattern
if (preg_match($pattern, $email)) {
return "Valid email.";
} else {
return "Invalid email format or blocked domain.";
}
}
Testing and debugging regex
Regex can get really complex, especially if you’re creating intricate patterns to include a lot of custom criteria. Small mistakes can result in your email validation not working as expected. Likewise, you could find that your regex validation works well for most email addresses, but not so well for edge cases.
Carrying out a good amount of testing will help you to see how your regex handles various scenarios, make adjustments where needed, and catch any errors. And this should be ongoing. Make sure you monitor and keep logs of any unusual responses or problematic emails so that you can review your regex against them.
There are free testing tools available that you can use to test your regex, Regex101 is a popular one.
Quick tips for using regex to validate emails
- Keep your patterns readable and maintainable. Try to avoid overly complex patterns and split the regex into smaller components where possible.
- Avoid overly strict validation rules to prevent valid emails from being blocked.
- Specify exactly which characters are allowed for each part of the email.
- Ensure compatibility across browsers/platforms. Different environments implement and process regex differently so make sure you test for consistent behavior.
- Block disposable email domains.
- Use non-capturing groups (unless you need parts of the email for later use) to avoid storing them and improve performance.
PHP email verification with an API
Using an email verification API instead of, or combined with, regex and native PHP functions gives you additional capabilities for an even cleaner database and better deliverability. It’s also pretty straightforward to implement—yes, you need to set up and implement the API, and it’s not free. But there’s no need for complex regex patterns, and the additional verification checks you get make it worth it.
As well as flagging email addresses that don’t follow the correct syntax, an email verification API can:
Identify emails with typos
Flag disposable domains automatically without manual configuration
Tell you if a mailbox is full and undeliverable
Perform real-time domain and mailbox checks to see if the mailbox actually exists
Plus some APIs, like MailerSend’s, can also tell you if an email address is role-based or belongs to a catch-all domain, so you can decide on the best way to handle every kind of email address.
Why does this matter? Well, all of these types of email addresses can result in lower engagement and higher bounce rates—which equals harm to your sender reputation and deliverability. If you use an email verification API to catch them at the point of signup, you’ll never make the mistake of sending to them. Plus, you’ll also get more accurate user numbers and less of a mess to clean up in your database.
How to set up PHP email verification with an API
Implementing an API to validate emails involves 3 steps:
1. Choosing your email verification service, installing the SDK and getting an API token.
2. Configuring the form (or other email collection point) on your website or app so emails can be sent to the backend for verification.
3. Configuring the backend so it receives the email address, sends it for verification, and returns the results.
First things first. You need an email verification API. If you’re a MailerSend user, you’re in luck. You’ll see in the app that there’s a built-in email verification tool, where you can verify a list of email addresses, and an API to go along with it.
Get 3,000 emails/month free
Sign up to MailerSend now to try the API and email verification and get a Hobby plan with 3,000 emails per month completely free.
Install the SDK and create an API token
Use the following command to install MailerSend’s official PHP SDK:
composer require mailersend/mailersend
In MailerSend, go to Integrations and click the Manage button in API tokens.
Click Create new token, enter a name and select your domain. Make sure you save your API key somewhere safe.
Learn more about creating and managing API tokens.
Configure the form on your app or website
Email addresses will be sent with Javascript to the backend (and then on to MailerSend) for verification. To configure this, you’ll need to change the <script> element of your HTML page.
< script >
document.getElementById('signupForm').addEventListener('submit', function(event) {
event.preventDefault(); // Prevent the form from submitting
const emailInput = document.getElementById('email');
const errorMessage = document.getElementById('error-message');
fetch('http://localhost/', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
email: emailInput.value
})
})
.then(response => response.json())
.then(result => {
console.log(result);
if (result.success) {
errorMessage.textContent = ''; // Clear any previous error message
alert('Email is valid! Form submitted.');
} else {
errorMessage.textContent = result.text || 'Please enter a valid email address.';
}
});
}); <
/script>
Configure your backend to perform the email verification
Add another script to the backend of your app or website so that it can retrieve the email address submission, send it to MailerSend for verification, and return the results so they can be displayed on the form.
This script example uses the MailerSend PHP SDK to make the API request and assumes you have a basic PHP environment set up with composer to manage dependencies as well as the phpdotenv package for environment variables. You should always use variables for sensitive data such as API keys.
<?php
require "vendor/autoload.php";
use MailerSend\MailerSend;
use Dotenv\Dotenv;
// Load environment variables from .env file
$dotenv = Dotenv::createImmutable(__DIR__);
$dotenv->load();
header("Access-Control-Allow-Origin: *");
header("Content-Type: application/json");
if ($_SERVER["REQUEST_METHOD"] === "POST") {
$input = json_decode(file_get_contents("php://input"), true);
$email = $input["email"] ?? "";
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo json_encode([
"success" => false,
"text" => "Invalid email format.",
"color" => "red",
]);
exit();
}
$apiKey = getenv("MAILERSEND_API_TOKEN"); // Get the API key from the environment variable
try {
$mailersend = new MailerSend(['api_key' => $apiKey ]);
$data = $mailersend->emailVerification->verifyEmail($email);
if (!empty($data["body"]["status"]) && $data["body"]["status"] === "valid") {
echo json_encode([
"success" => true,
"text" => "Email is valid.",
"color" => "green",
]);
} else {
echo json_encode([
"success" => false,
"text" => "Email is invalid.",
"color" => "red",
]);
}
} catch (Exception $e) {
error_log("Error: " . $e->getMessage());
echo json_encode([
"success" => false,
"text" => "An error occurred during validation.",
"color" => "red",
]);
}
} else {
echo json_encode([
"success" => false,
"text" => "Invalid request method.",
"color" => "red",
]);
}
?>
You’ll notice that this script includes filter_var() to validate the email address format before passing it on the API. Combining methods in this way helps to increase the accuracy of your email address validation.
Combining an API with regex
You might find that combining an API and regex for a layered approach works better for your use case. For example, you could use regex to filter out or only allow specific domains and only submit those that meet your pattern's conditions for verification while rejecting the rest, preserving your API and verification quota.
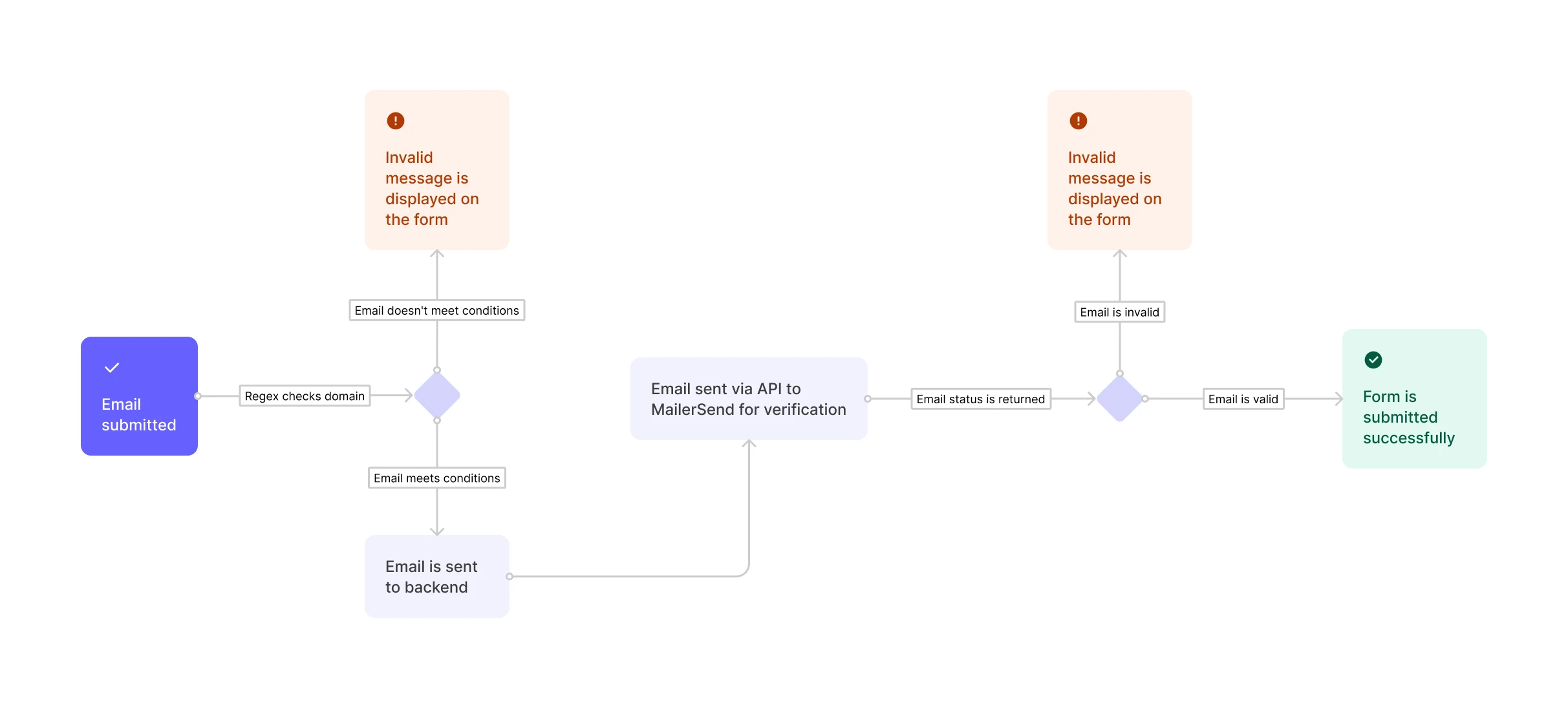
Validating emails with PHP in bulk
If you want to validate a list of email addresses in a database at regular intervals, you can use a cron job to schedule the validation checks and log the results in your database.
<?php
require "vendor/autoload.php";
use MailerSend\MailerSend;
use Dotenv\Dotenv;
use PDO;
// Load environment variables from .env file
$dotenv = Dotenv::createImmutable(__DIR__);
$dotenv->load();
$apiKey = getenv("MAILERSEND_API_TOKEN");
$dbHost = getenv("DB_HOST");
$dbName = getenv("DB_NAME");
$dbUser = getenv("DB_USER");
$dbPass = getenv("DB_PASS");
// Check if API key is set
if (!$apiKey) {
error_log("Error: Missing MailerSend API key.");
exit();
}
// Connect to the database
try {
$pdo = new PDO("mysql:host=$dbHost;dbname=$dbName;charset=utf8mb4", $dbUser, $dbPass, [
PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION,
]);
} catch (Exception $e) {
error_log("Database connection failed: " . $e->getMessage());
exit();
}
// Fetch emails that need validation
$query = "SELECT id, email FROM users WHERE email_verified = 0 LIMIT 100"; // Adjust limit as needed
$statement = $pdo->prepare($query);
$statement->execute();
$emails = $statement->fetchAll(PDO::FETCH_ASSOC);
if (!$emails) {
error_log("No emails to validate.");
exit();
}
$client = new Client();
foreach ($emails as $user) {
$id = $user["id"];
$email = $user["email"];
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Mark as invalid in the database
$pdo->prepare("UPDATE users SET email_valid = 0 WHERE id = ?")->execute([$id]);
error_log("Invalid format: $email");
continue;
}
try {
$mailersend = new MailerSend(['api_key' => $apiKey ]);
$response = $mailersend->emailVerification->verifyEmail($email);
$data = $response["body"];
$status = $data["status"] === "valid" ? 1 : 0;
// Update database with verification result
$pdo->prepare("UPDATE users SET email_valid = ? WHERE id = ?")->execute([$status, $id]);
error_log("Checked: $email -> " . ($status ? "Valid" : "Invalid"));
} catch (Exception $e) {
error_log("Validation error for $email: " . $e->getMessage());
}
}
error_log("Email validation process completed.");
?>
To run the script, you’ll need to add this line to your cron jobs:
0 17 * * * /usr/bin/php /path/to/bulk_email_validation.php >> /var/log/email_validation.log 2>&1
This schedules the script to run every day at 5pm.
Email validation matters… a lot
Signup forms without email validation let just about anyone or anything into your app—it’s a bit like leaving an open portal to chaos. It impacts deliverability, user experience, and security… and it’s just bad for business!
Deliverability
There are several ways invalid emails can harm deliverability, including:
Increased bounce rates: If the email address used to sign up doesn’t exist, it will bounce, and it will bounce hard. Hard and soft bounces are to be expected in small numbers, but if your bounce rate creeps up too high, mailbox providers like Gmail and Outlook will interpret this as you carrying out bad sending practices or being a spammer. This will result in your sender reputation being impacted and your emails being blocked or marked as spam.
Being added to a blocklist: If you keep sending to nonexistent email addresses, you could get your IP address or domain blocklisted. If this happens, it’s likely that your emails will be blocked by ISPs until you get removed from the list, which can take a lot of time and effort.
Decreased engagement: If you send emails to a large number of invalid or fake email addresses, it’s going to heavily skew your metrics. You’ll get lower open and click rates, which not only impacts your reporting but also tells ISPs that the people you’re sending to aren’t interested in what you have to say.
User experience
You want your user experience to be as frictionless as possible. If users enter an invalid email address by mistake, they won’t be able to receive relevant messages. This can lead to a lot of frustration and leave a bad impression of your website or app. Imagine:
Never receiving welcome emails or email verification messages
Not receiving other important emails like order confirmations or billing notifications
Having difficulty managing account settings
Not being able to access your account if you get logged out and didn’t realise you entered the wrong email address
Failed password reset requests due to the wrong email address being associated with the account
A lot of us would give up after the second try and head straight for the nearest competitor. Simple email validation can prevent all this.
Security
Accepting invalid emails on your signup forms can lead to compromised data, bot attacks, phishing attacks, and abuse of your platform. Here’s how:
Access to a user’s account and/or data: If someone receives an email because a user entered their email address mistakenly instead of their own, they’ll receive the user’s emails and could gain access to their account. Now, they could be a good person who ignores and deletes the messages, or they could be a bad actor who sees an opportunity. Like Forrest Gump said, “Life is like a box of chocolates, you never know what you’re gonna get”. So it’s better to remove the possibility by validating on sign up.
Bot attacks and abuse: Bots can be used for mass account creation using disposable email addresses. While they can target businesses to inflate user numbers and waste resources, they can also be used to abuse platforms that offer free trials or credit, referral rewards, and discounts, as well as for spam and fraud. DoS (Denial of Service) attacks are another type of attack carried out by bots.
Business and marketing initiatives
Increased costs, missed opportunities, and reputational damage—a recipe for disaster brought to you by a lack of email address validation. If you find yourself with a database full of invalid emails and bots, it will have a knock-on effect across your business’ overall strategy and initiatives.
Wasted money and resources: It costs money to send emails at scale, and if you send to a large number of invalid addresses, then you might end up paying way more than you should be. It can also result in API requests and other sending limits being met more quickly.
Missed opportunities: If customers aren’t receiving your emails—either because they entered the wrong email address or because your deliverability has taken a hit—they won’t take any of the actions that you want from your messages. Whether that’s an abandoned cart email, cross-sell, or crucial update to their billing information.
Misinformed decision making: Accurate data is key to understanding your customers and making strategic marketing and business decisions. If that data is inaccurate, it can make it harder to optimize your operations or result in poor decisions.
Reputational damage: If the presence of invalid emails begins to impact the user experience or put your users and their data at risk, it will lead to a lack of trust and potential penalties from relevant regulatory authorities.
Skipping email validation? Think twice
Email validation isn’t just a nice-to-have feature—it should be a fundamental element of your app or website. Whether you use simple PHP functions, regex, an API, or some combo of methods, it’s a simple process that can save you a lot of time (and tears) cleaning up databases, fixing deliverability issues, and addressing user experience mishaps.
An email verification API will give you the most comprehensive checks, so that will always be our go-to recommendation. You can sign up to MailerSend for free and check out all of our features, from email sending via SMTP and API and SMS, to inbound message processing and email verification.
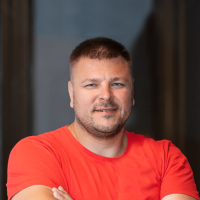