Scalable transactional email solution for agencies
Simplify agency email management for multiple clients by running them on a single account. Keep domains separate, customize access, and create uniquely branded templates.
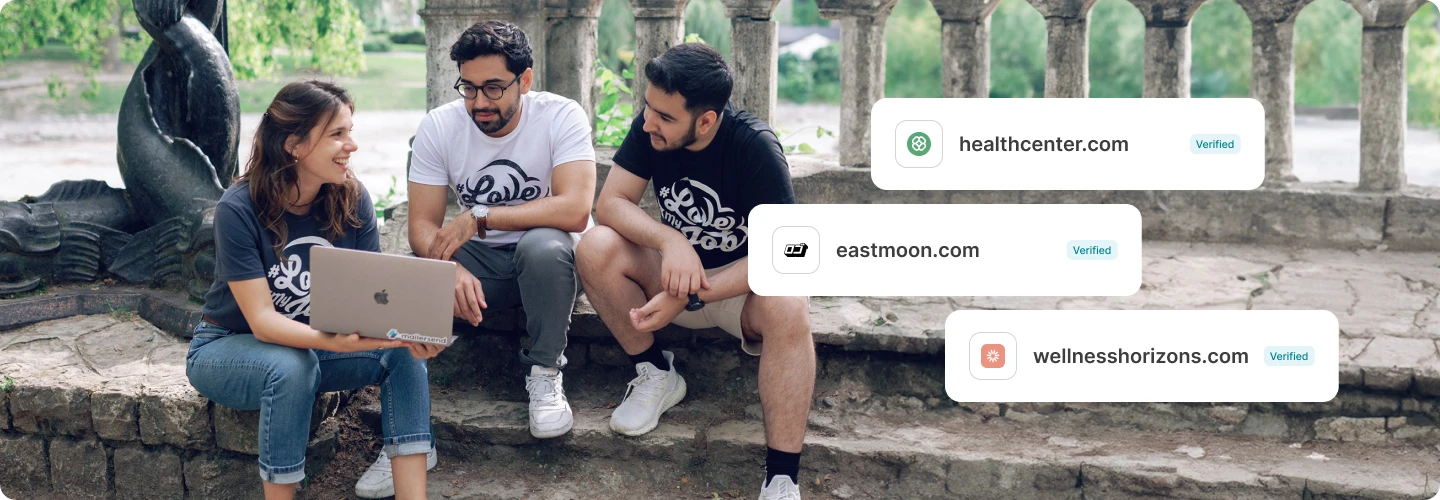
SDKs to fit every app or website
curl -X POST \
https://api.mailersend.com/v1/email \
-H 'Content-Type: application/json' \
-H 'X-Requested-With: XMLHttpRequest' \
-H 'Authorization: Bearer {place your token here without brackets}' \
-d '{
"from": {
"email": "your@email.com"
},
"to": [
{
"email": "your@client.com"
}
],
"subject": "Hello from MailerSend!",
"text": "Greetings from the team, you got this message through MailerSend.",
"html": "Greetings from the team, you got this message through MailerSend.
"
}'
import { MailerSend, EmailParams, Sender, Recipient } from "mailersend";
const mailerSend = new MailerSend({
api_key: "key",
});
const sentFrom = new Sender("you@yourdomain.com", "Your name");
const recipients = [
new Recipient("your@client.com", "Your Client")
];
const emailParams = new EmailParams()
.setFrom(sentFrom)
.setTo(recipients)
.setReplyTo(sentFrom)
.setSubject("This is a Subject")
.setHtml("This is the HTML content")
.setText("This is the text content");
await mailerSend.email.send(emailParams);
$mailersend = new MailerSend();
$recipients = [
new Recipient('your@client.com', 'Your Client'),
];
$emailParams = (new EmailParams())
->setFrom('your@email.com')
->setFromName('Your Name')
->setRecipients($recipients)
->setSubject('Subject')
->setHtml('Greetings from the team, you got this message through MailerSend.')
->setText('Greetings from the team, you got this message through MailerSend.');
$mailersend->email->send($emailParams);
php artisan make:mail ExampleEmail
Mail::to('you@client.com')->send(new ExampleEmail());
from mailersend import emails
mailer = emails.NewEmail()
mail_body = {}
mail_from = {
"name": "Your Name",
"email": "your@domain.com",
}
recipients = [
{
"name": "Your Client",
"email": "your@client.com",
}
]
mailer.set_mail_from(mail_from, mail_body)
mailer.set_mail_to(recipients, mail_body)
mailer.set_subject("Hello!", mail_body)
mailer.set_html_content("Greetings from the team, you got this message through MailerSend.
", mail_body)
mailer.set_plaintext_content("Greetings from the team, you got this message through MailerSend.", mail_body)
mailer.send(mail_body)
require "mailersend-ruby"
# Intialize the email class
ms_email = Mailersend::Email.new
# Add parameters
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_recipients("email" => "your@client.com", "name" => "Your Client")
ms_email.add_from("email" => "your@domain.com", "name" => "Your Name")
ms_email.add_subject("Hello!")
ms_email.add_text("Greetings from the team, you got this message through MailerSend.")
ms_email.add_html("Greetings from the team, you got this message through MailerSend.")
# Send the email
ms_email.send
package main
import (
"context"
"os"
"fmt"
"time"
"github.com/mailersend/mailersend-go"
)
func main() {
// Create an instance of the mailersend client
ms := mailersend.NewMailersend(os.Getenv("MAILERSEND_API_KEY"))
ctx := context.Background()
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
subject := "Subject"
text := "This is the text content"
html := "This is the HTML content
"
from := mailersend.From{
Name: "Your Name",
Email: "your@domain.com",
}
recipients := []mailersend.Recipient{
{
Name: "Your Client",
Email: "your@client.com",
},
}
// Send in 5 minute
sendAt := time.Now().Add(time.Minute * 5).Unix()
tags := []string{"foo", "bar"}
message := ms.Email.NewMessage()
message.SetFrom(from)
message.SetRecipients(recipients)
message.SetSubject(subject)
message.SetHTML(html)
message.SetText(text)
message.SetTags(tags)
message.SetSendAt(sendAt)
message.SetInReplyTo("client-id")
res, _ := ms.Email.Send(ctx, message)
fmt.Printf(res.Header.Get("X-Message-Id"))
}
import com.mailersend.sdk.Email;
import com.mailersend.sdk.MailerSend;
import com.mailersend.sdk.MailerSendResponse;
import com.mailersend.sdk.exceptions.MailerSendException;
public void sendEmail() {
Email email = new Email();
email.setFrom("name", "your email");
email.addRecipient("name", "your@recipient.com");
// you can also add multiple recipients by calling addRecipient again
email.addRecipient("name 2", "your@recipient2.com");
// there's also a recipient object you can use
Recipient recipient = new Recipient("name", "your@recipient3.com");
email.AddRecipient(recipient);
email.setSubject("Email subject");
email.setPlain("This is the text content");
email.setHtml("This is the HTML content
");
MailerSend ms = new MailerSend();
ms.setToken("Your API token");
try {
MailerSendResponse response = ms.emails().send(email);
System.out.println(response.messageId);
} catch (MailerSendException e) {
e.printStackTrace();
}
}
Go low/no-code with SMTP
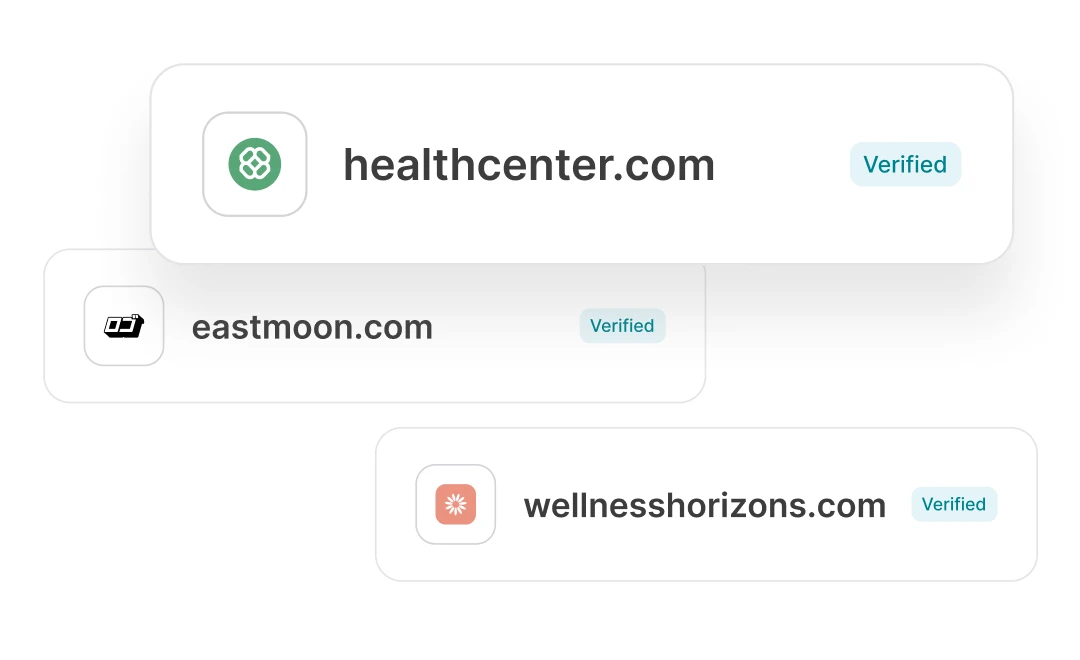
One account, multiple domains
Use a single account to add, manage, and send from each client’s unique domain while keeping all settings, data, API tokens, and templates separate.
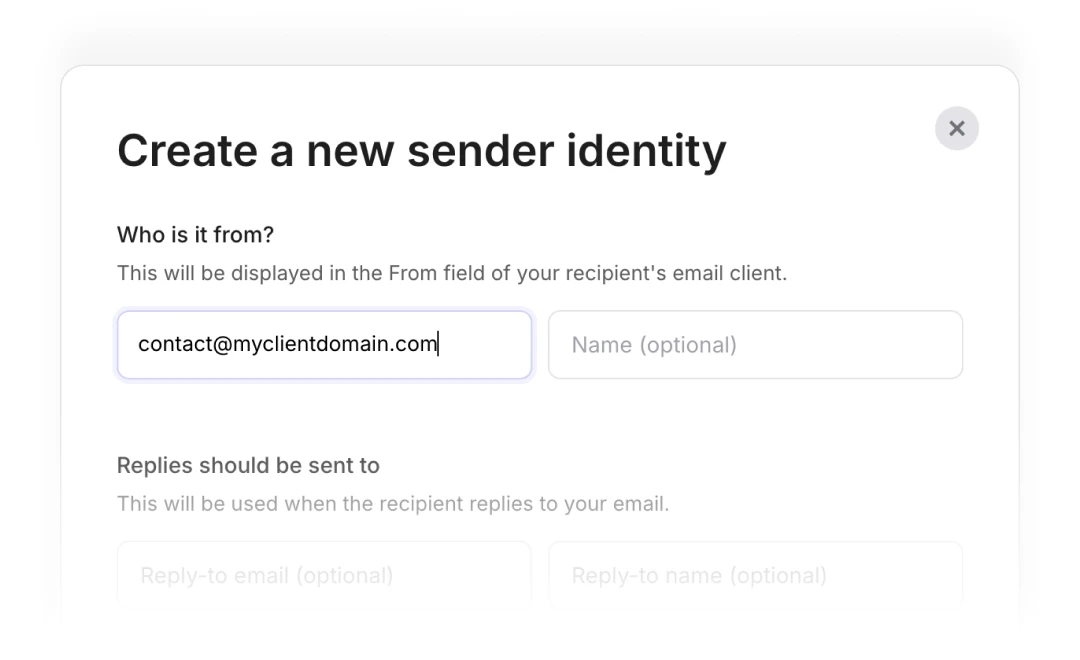
Skip domain verification with sender identities
No access to your customer’s domain? No problem. Send emails for them—from them—with sender identities. Simply add the client’s email address you want to send from, have them verify it, and you’re all set.
Keep clients in the loop with custom access permissions
Super precise access controls enable you to invite clients to view or manage their emails without gaining access to other clients’ domains, settings, and more. Give as little or as much access as you need.
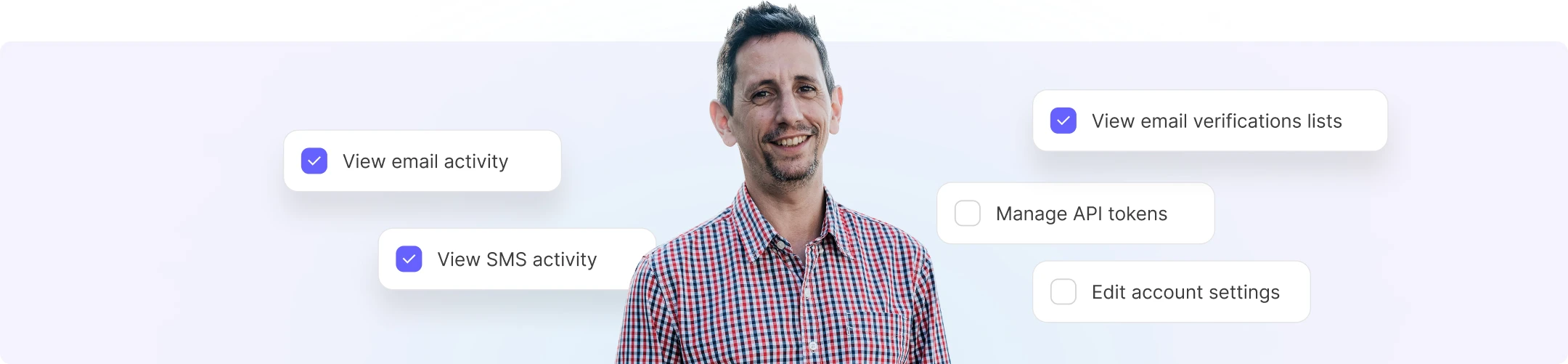
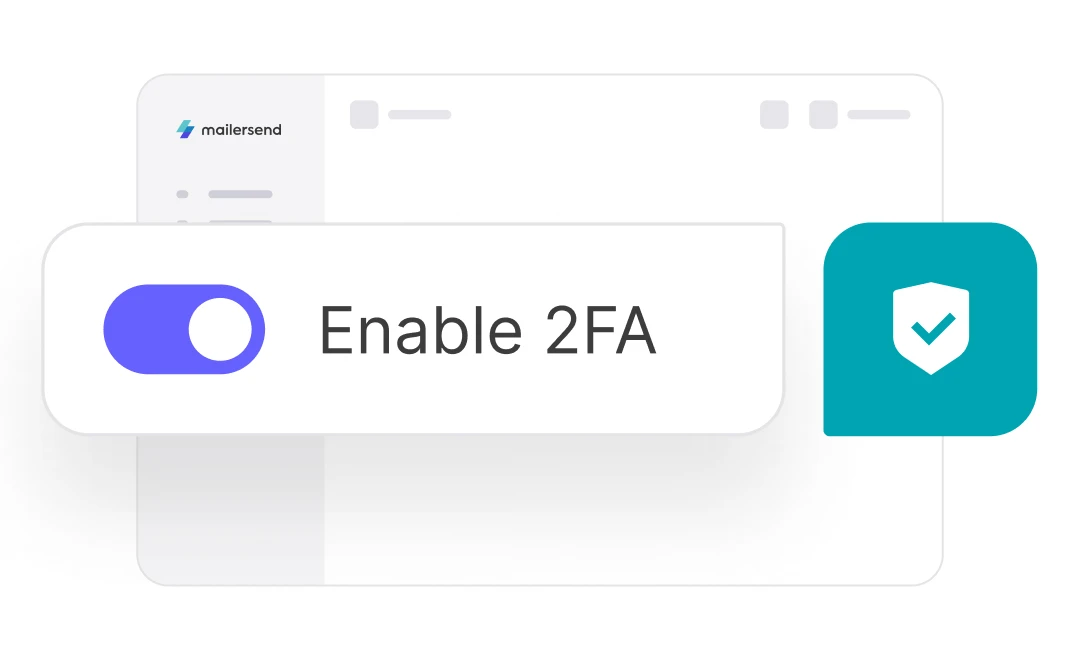
Maintain account security
Enable 2FA for all account users to add an extra layer of protection and add approved IP addresses to your IP allowlist to prevent unauthorized sending activity.
Build branded emails with 3 user-oriented builders
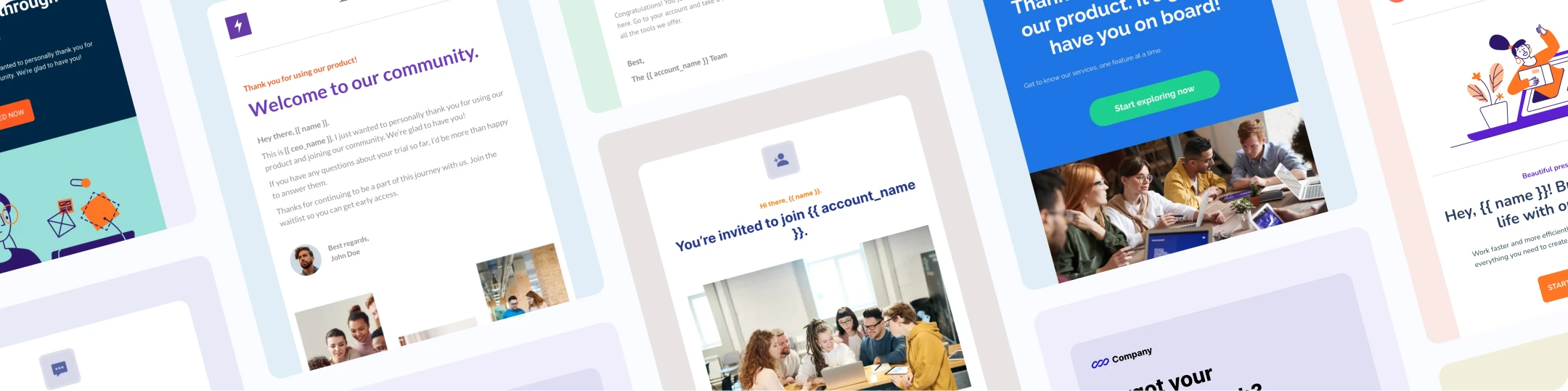
Drag & drop editor
Create professionally designed, responsive emails with 90+ pre-built blocks for content, interactive design elements, e-commerce, social, and more.
HTML editor
Import and edit existing HTML templates or build new ones from scratch. Speed up template creation with snippets and error tagging.
Rich-text email editor
Make plain-text emails more professional and engaging with HTML formatting that you can drop right in without coding a thing.
Endless ways to optimize clients’ digital strategy
-
E-commerce
Send professional, personalized order confirmations, shipping updates, delivery confirmations, and more. -
Bars, restaurants & hotels
Improve online reservations with booking and cancellation confirmation emails, notifications, and review requests. -
Health, beauty & wellness
Confirm appointments, send reminders, inform customers about important information, and provide an easy way to change or cancel.
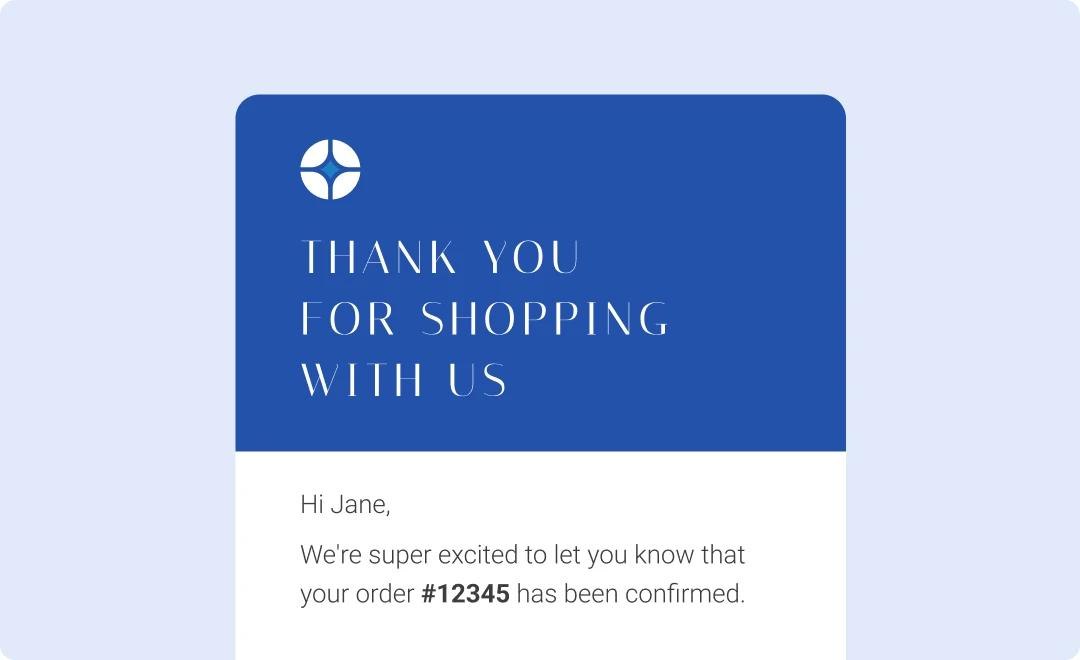
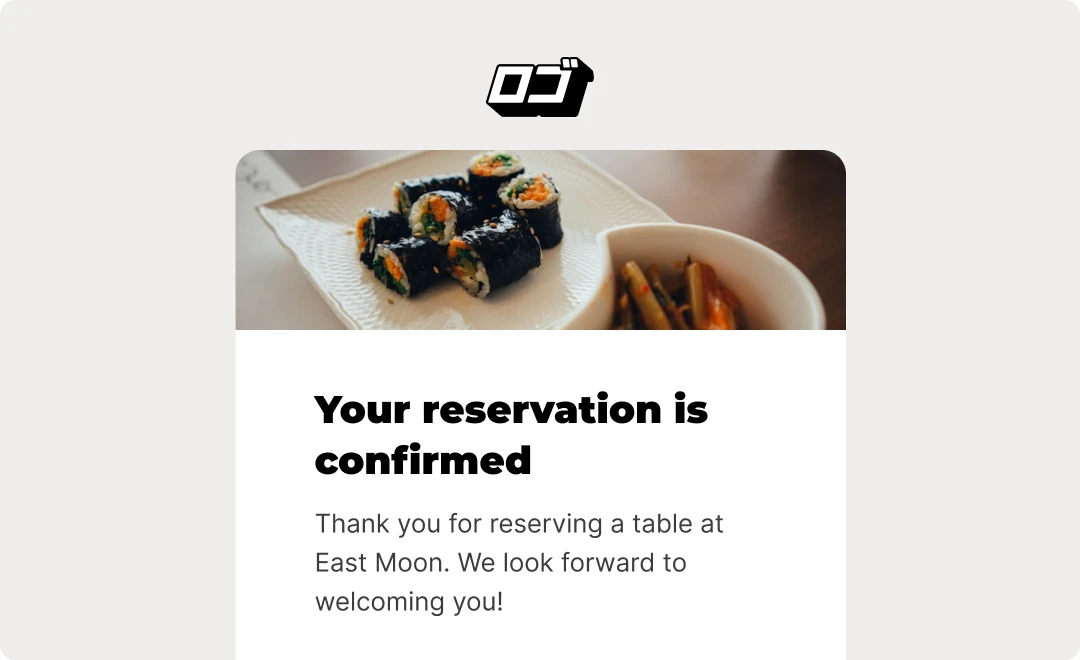
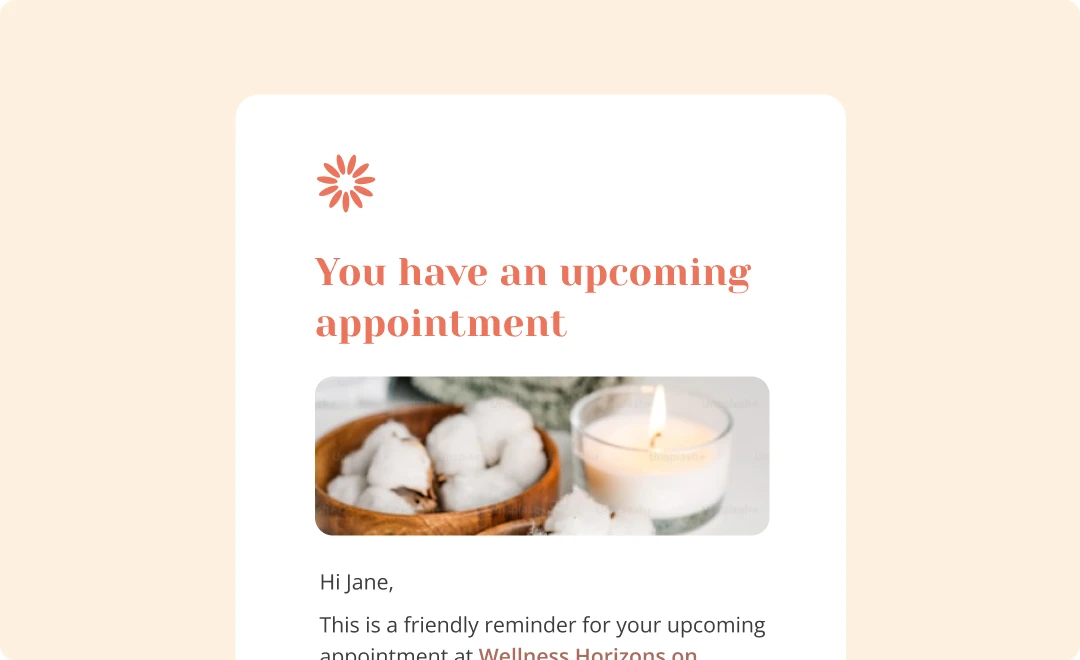
Monitor clients’ sending activity & performance
Webhook notifications
Receive webhook notifications about email activity to trigger workflows in connected apps or get real-time alerts about critical events.
Real-time activity dashboard
Follow each email’s journey in the app and filter activity by domain to learn how your clients’ transactional emails are performing.
In-depth analytics
Access a wide range of key metrics and track important KPIs. Create custom reports you can share with your clients to keep them in the loop.
Integrations
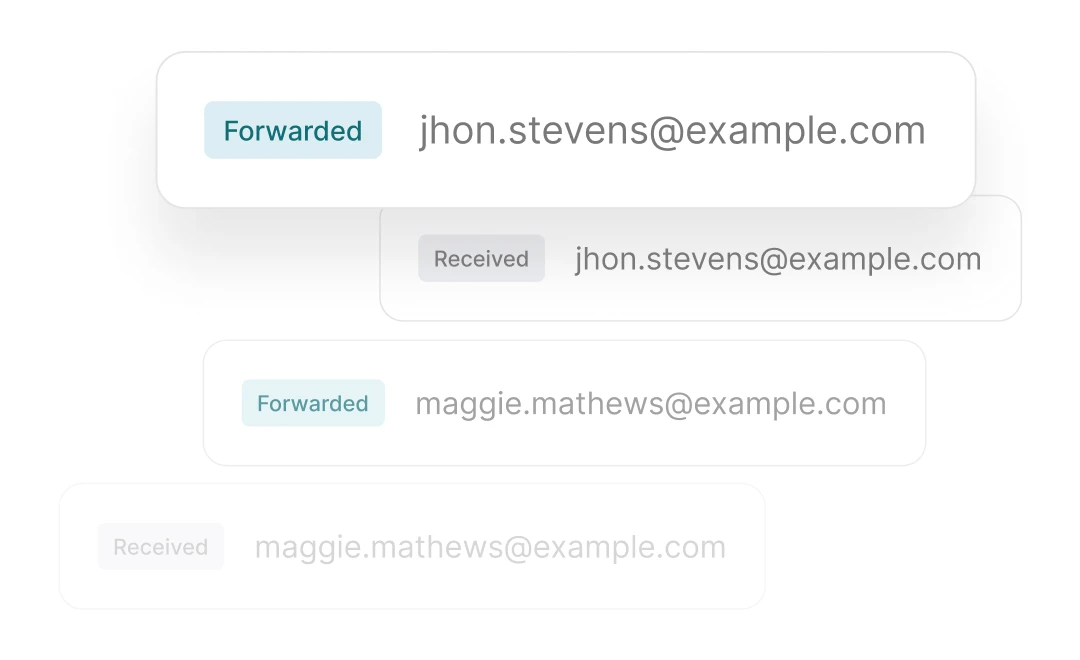
Enable two-way communication
Create inbound routes to process and parse incoming emails to clients’ apps, websites, or support platforms. Optimize support ticketing, let users reply to messages and comments, and add extra email-based functionality.

Provide an omnichannel solution with SMS
Help your clients reach more customers by sending transactional SMS messages alongside their email. Use the SMS API to send 2FA messages, shipping notifications, reminders, and more.
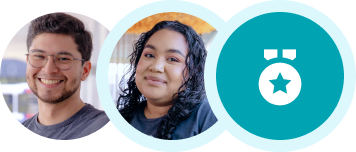
24/7 award-winning support
No bots, only humans! Whether you need help getting started, setting up advanced features, or troubleshooting a technical issue, our friendly customer support team is always happy to help.
A cost-effective solution with advanced tools for your agency
Hobby
Starter
Professional
Enterprise
Frequently Asked Questions
How does MailerSend ensure high email deliverability for agencies managing multiple clients?
With multiple domains, you can keep sending activity separate for each client, ensuring that domain reputation is unaffected by the activity of another.
What types of agencies benefit most from using MailerSend?
If you are a digital marketing agency, design agency, or web solutions agency providing whole digital solutions for clients, your agency can benefit from using MailerSend due to its team-friendly interface and features, granular user roles, and simplified integration.
What level of customer support does MailerSend provide for agencies?
All paid accounts have access to email and live chat support, while Professional and Enterprise accounts also have priority support. Rest assured, no matter which plan you choose, our support team is friendly, knowledgeable, and quick to respond.
Can MailerSend handle the volume of emails needed for large-scale agency campaigns?
Yes, MailerSend is the ideal email API for agencies, built on a power infrastructure optimized for high volumes of transactional emails and account scalability.
How easy is it to onboard new clients onto MailerSend?
You can quickly add and verify your clients’ domains or add sender identities and use our SDKs to integrate email sending for the app or website. You can also easily give clients access by creating custom roles and restricting which domains, features, and settings they can see, keeping each client’s emails separate and making user management easy. What’s more, the MailerSend app is extremely intuitive, and we have a wealth of help guides to get you started!