Hassle-free bulk SMS API
Send and receive high volumes of transactional text messages with MailerSend’s simple yet robust bulk SMS API service.
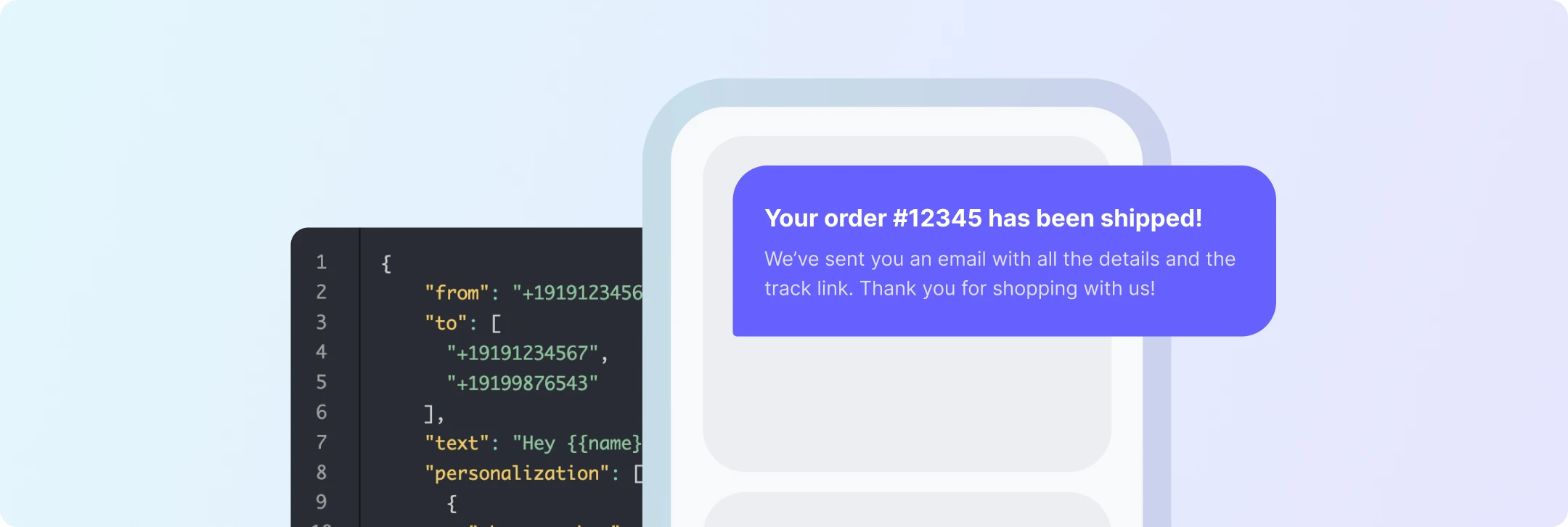
High deliverability
Direct network integration and SMS prioritization ensure quick, consistent delivery over mobile networks.
Increased engagement
Reach more customers with the 98% open rates of SMS and enhance your brand experience.
Cost efficient
A cost-effective way to communicate with recipients thanks to the low cost per text and high open rates.
Developer-friendly bulk SMS gateway API integration
curl -X POST \
https://api.mailersend.com/v1/sms \
-H 'Content-Type: application/json' \
-H 'X-Requested-With: XMLHttpRequest' \
-H 'Authorization: Bearer {place your token here without brackets}' \
-d '{
"from": "+18332552485",
"to": [
"+12345678900",
],
"text": "This is just a friendly hello"
}'
"use strict";
require('dotenv').config()
const { MailerSend, SMSParams } = require("mailersend");
const mailersend = new MailerSend({
apiKey: process.env.API_KEY,
});
const recipients = [
"+18332647501"
];
const smsParams = new SMSParams()
.setFrom("+18332647501")
.setRecipients(recipients)
.setText("This is the text content");
mailersend.sendSms(smsParams);
use MailerSend\MailerSend;
use MailerSend\Helpers\Builder\SmsParams;
$mailersend = new MailerSend(['api_key' => 'key']);
$smsParams = (new SmsParams())
->setFrom('+12065550101')
->setTo(['+12065550102'])
->addRecipient('+12065550103')
->setText('Text');
$sms = $mailersend->sms->send($smsParams);
from mailersend import sms_sending
from dotenv import load_dotenv
load_dotenv()
mailer = sms_sending.NewSmsSending(os.getenv('MAILERSEND_API_KEY'))
# Number belonging to your account in E164 format
number_from = "+11234567890"
# You can add up to 50 recipient numbers
numbers_to = [
"+11234567891",
"+11234567892"
]
text = "Hi {{name}} how are you?"
personalization = [
{
"phone_number": "+11234567891",
"data": {
"name": "Mike"
}
},
{
"phone_number": "+11234567892",
"data": {
"name": "John"
}
}
]
print(mailer.send_sms(number_from, numbers_to, text, personalization))
require "mailersend-ruby"
# Intialize the SMS class
ms_sms = Mailersend::SMS.new
# Add parameters
ms_sms.add_from('your-number')
ms_sms.add_to('client-number')
ms_sms.add_text('This is the message content')
personalization = {
phone_number: 'client-number',
data: {
test: 'Test Value'
}
}
ms_sms.add_personalization(personalization)
# Send the SMS
ms_sms.send
package main
import (
"context"
"os"
"fmt"
"time"
"github.com/mailersend/mailersend-go"
)
func main() {
// Create an instance of the mailersend client
ms := mailersend.NewMailersend(os.Getenv("MAILERSEND_API_KEY"))
ctx := context.Background()
ctx, cancel := context.WithTimeout(ctx, 5*time.Second)
defer cancel()
message := ms.Sms.NewMessage()
message.SetFrom("your-number")
message.SetTo([]string{"client-number"})
message.SetText("This is the message content {{ var }}")
personalization := []mailersend.SmsPersonalization{
{
PhoneNumber: "client-number",
Data: map[string]interface{}{
"var": "foo",
},
},
}
message.SetPersonalization(personalization)
res, _ := ms.Sms.Send(context.TODO(), message)
fmt.Printf(res.Header.Get("X-SMS-Message-Id"))
}
import com.mailersend.sdk.MailerSend;
import com.mailersend.sdk.exceptions.MailerSendException;
public void sendSms() {
MailerSend ms = new MailerSend();
ms.setToken("mailersend token");
try {
String messageId = ms.sms().builder().from("from phone number")
.addRecipient("to phone number")
.text("test sms {{name}}")
.addPersonalization("to phone number", "name", "name personalization")
.send();
System.out.println(messageId);
} catch (MailerSendException e) {
e.printStackTrace();
}
}
Low/no-code integrations with 1000’s of apps
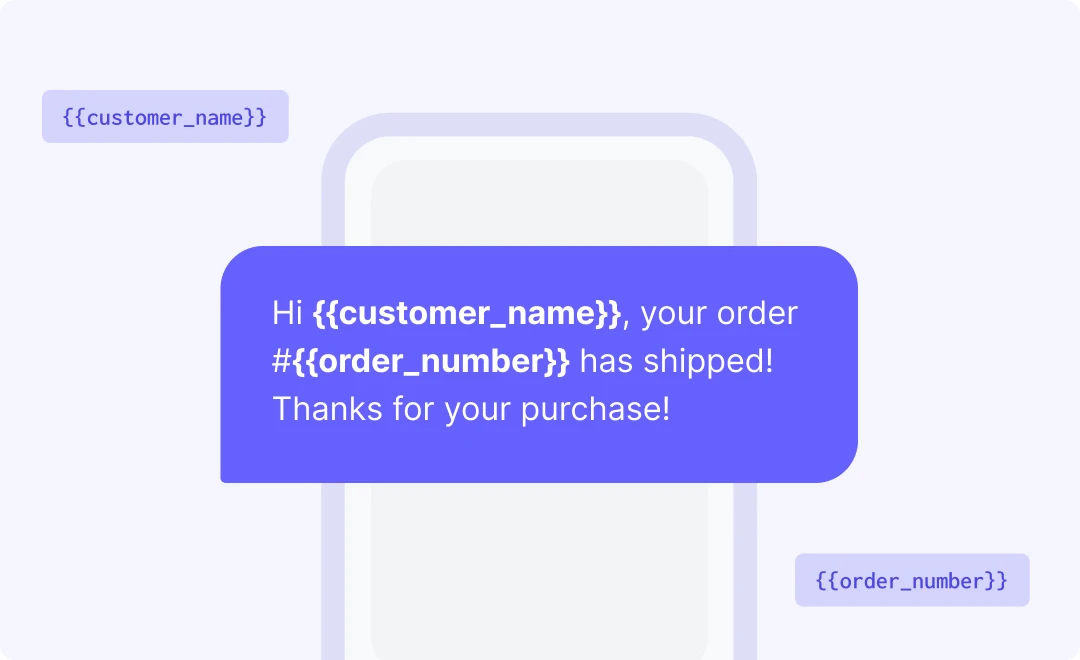
Personalization made easy
Seamlessly insert customer information with variables for personalized bulk SMS messaging that engages users. Add customers’ names, order details, and more.
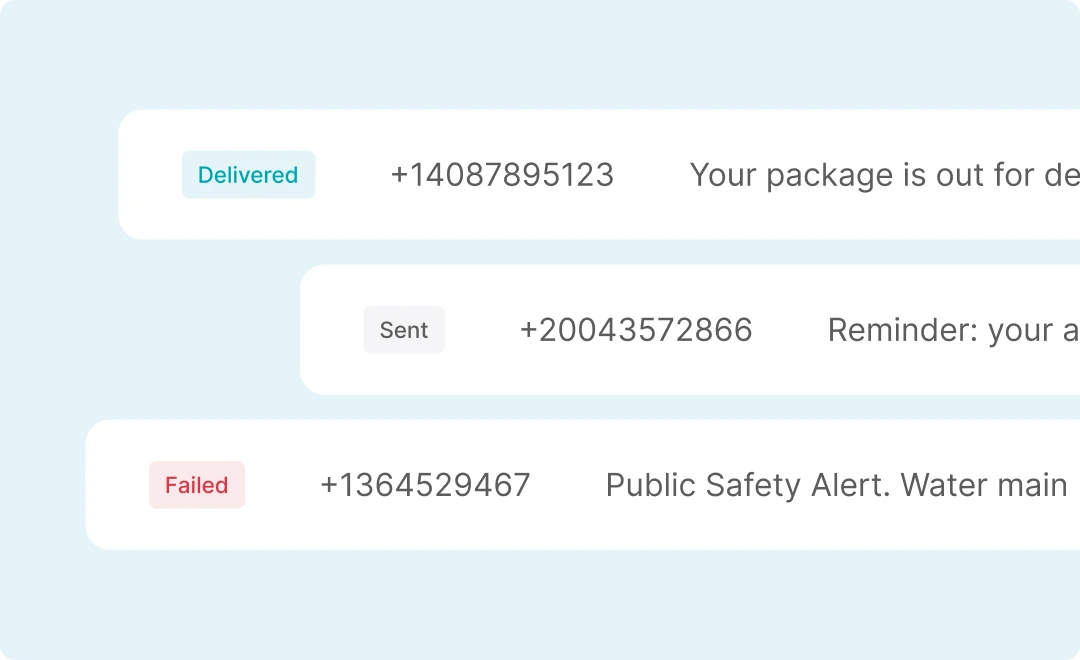
Bulk SMS activity tracking
Monitor the status of your SMS text messages to gauge performance and make adjustments. See when a message is processed, queued, sent, delivered, or failed.
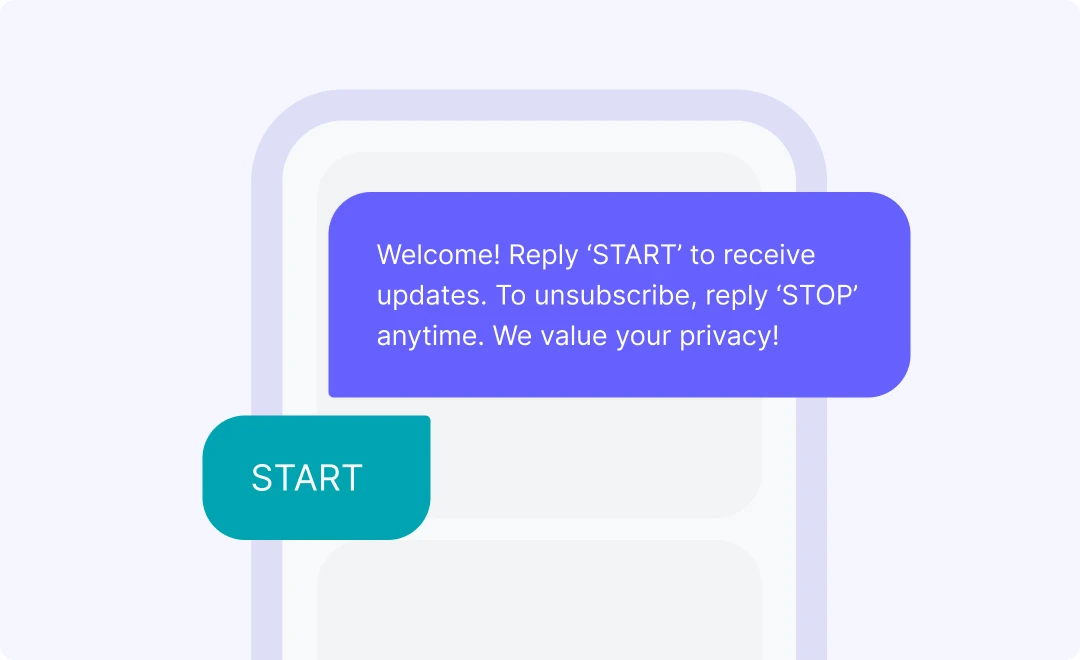
Efficient subscriber management
Automate the opt-in/opt-out process with “Start” and “Stop” SMS commands. Enhance the user experience by giving control to recipients and make managing suppressions easy.
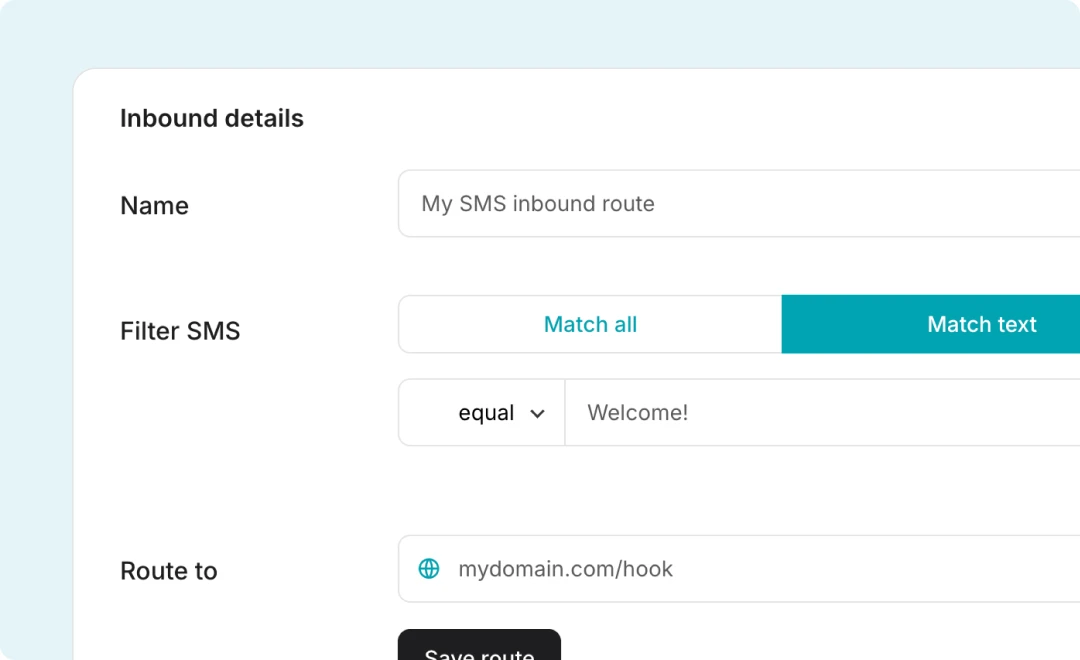
Simple inbound SMS processing
Use inbound routing to parse incoming SMS messages to your CRM or other apps. Let customers and users communicate with you via text for support, information requests, appointment confirmations, and more.
Endless ways to use bulk SMS API
-
SaaS use cases
Send SaaS security alerts, OTPs (One-time passwords), 2FA (two-factor authentication), billing notifications and more. -
Banking & fintech use cases
Keep users informed with banking SMS messages including transaction notifications, payment reminders, and fraud alerts. -
E-commerce use cases
Provide a second-to-none experience with e-commerce SMS such as shipping notifications, refund updates, surveys and more. -
Government & public services use cases
Engage and inform the community with emergency alert text messages, public health announcements, and event reminders. -
Healthcare use cases
Improve patient care with appointment reminders and confirmations, test result notifications, and important news.
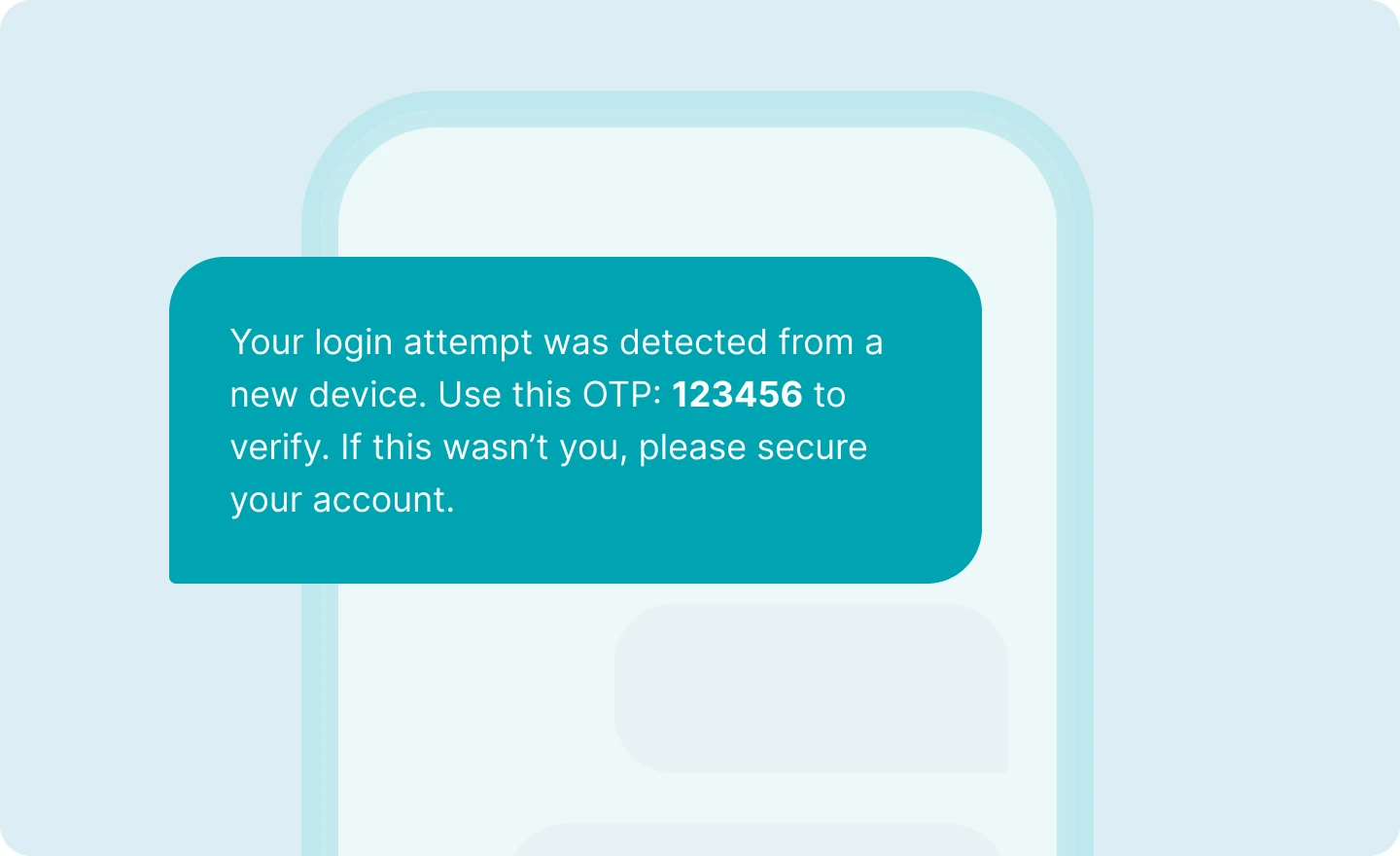
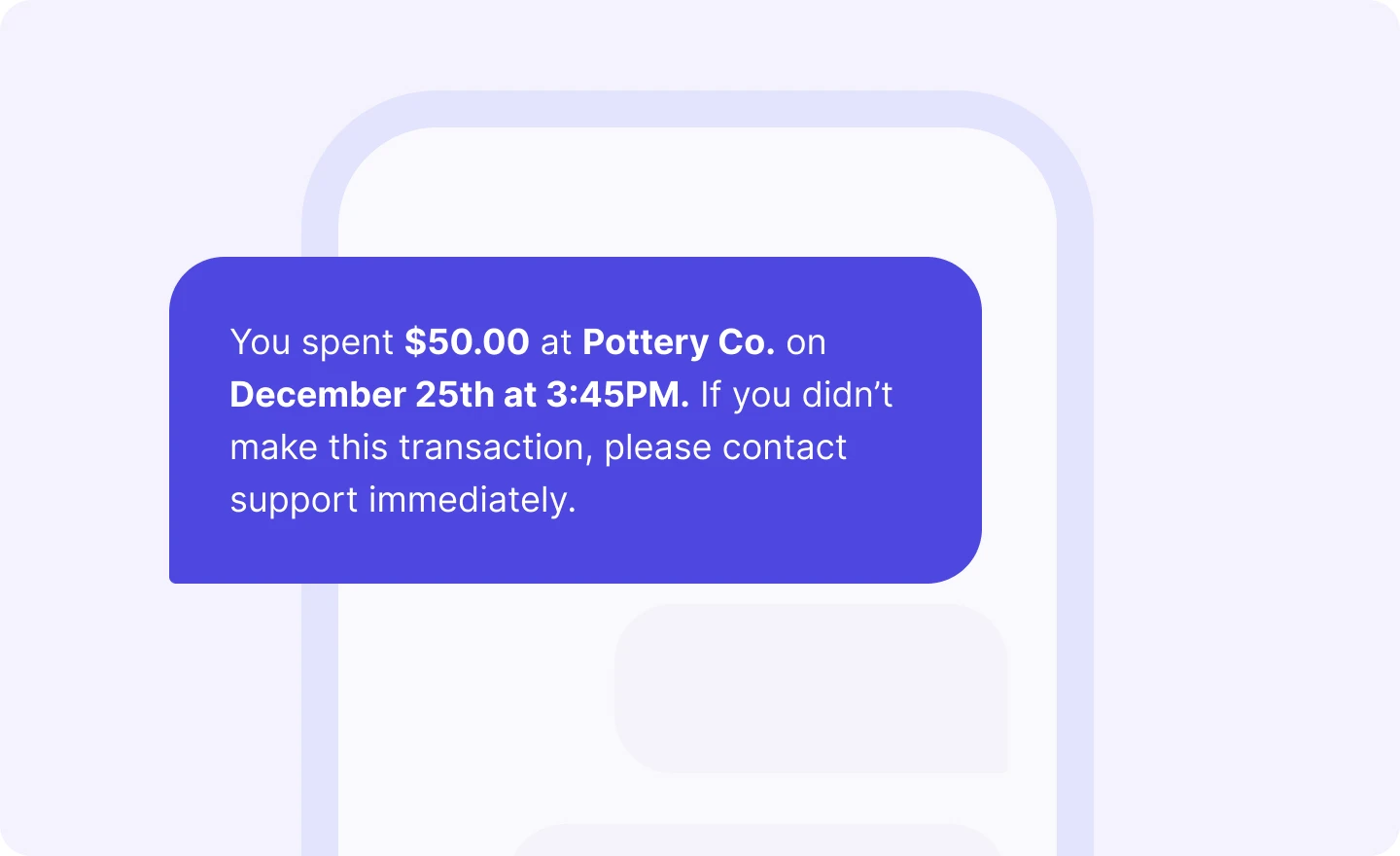
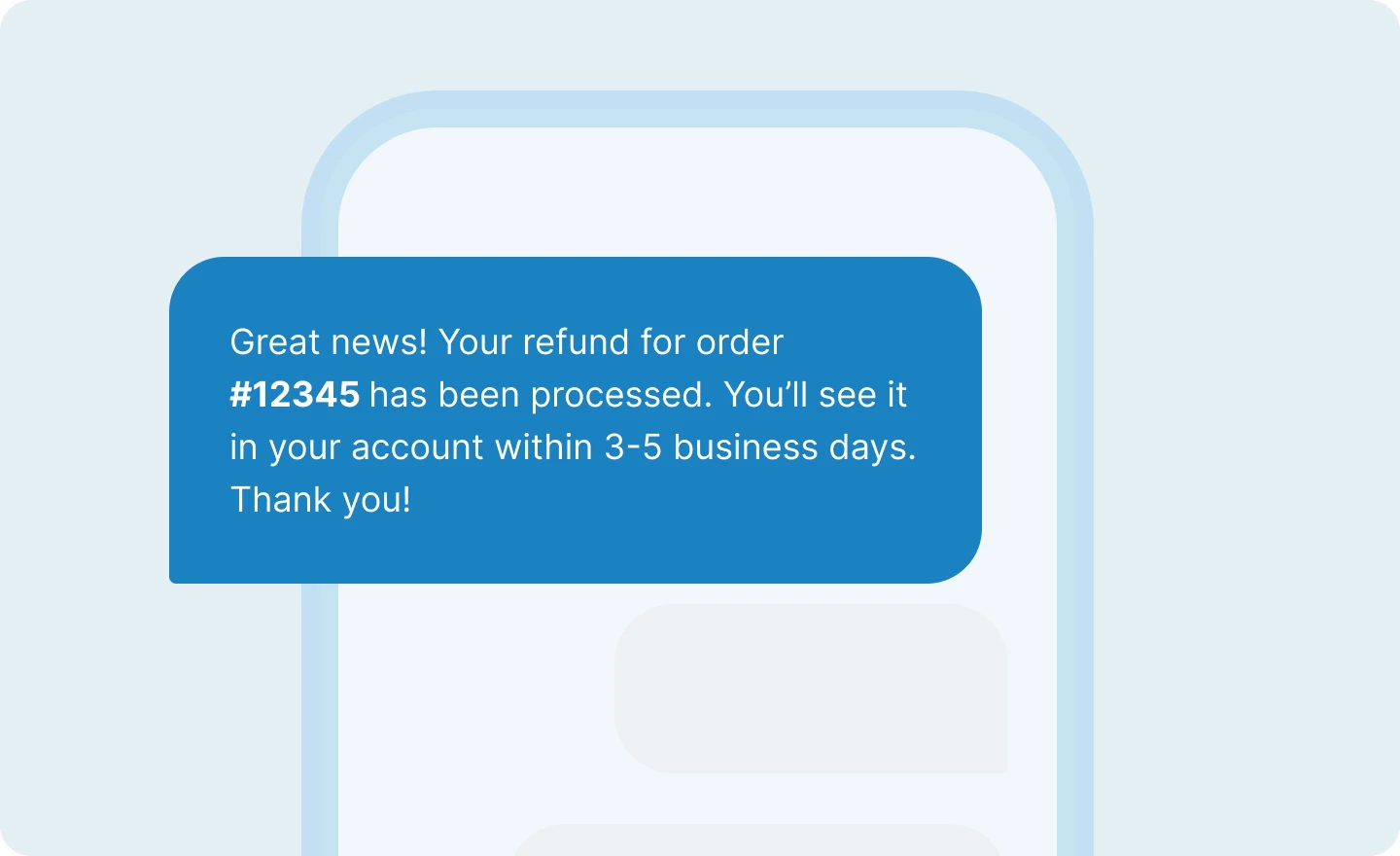
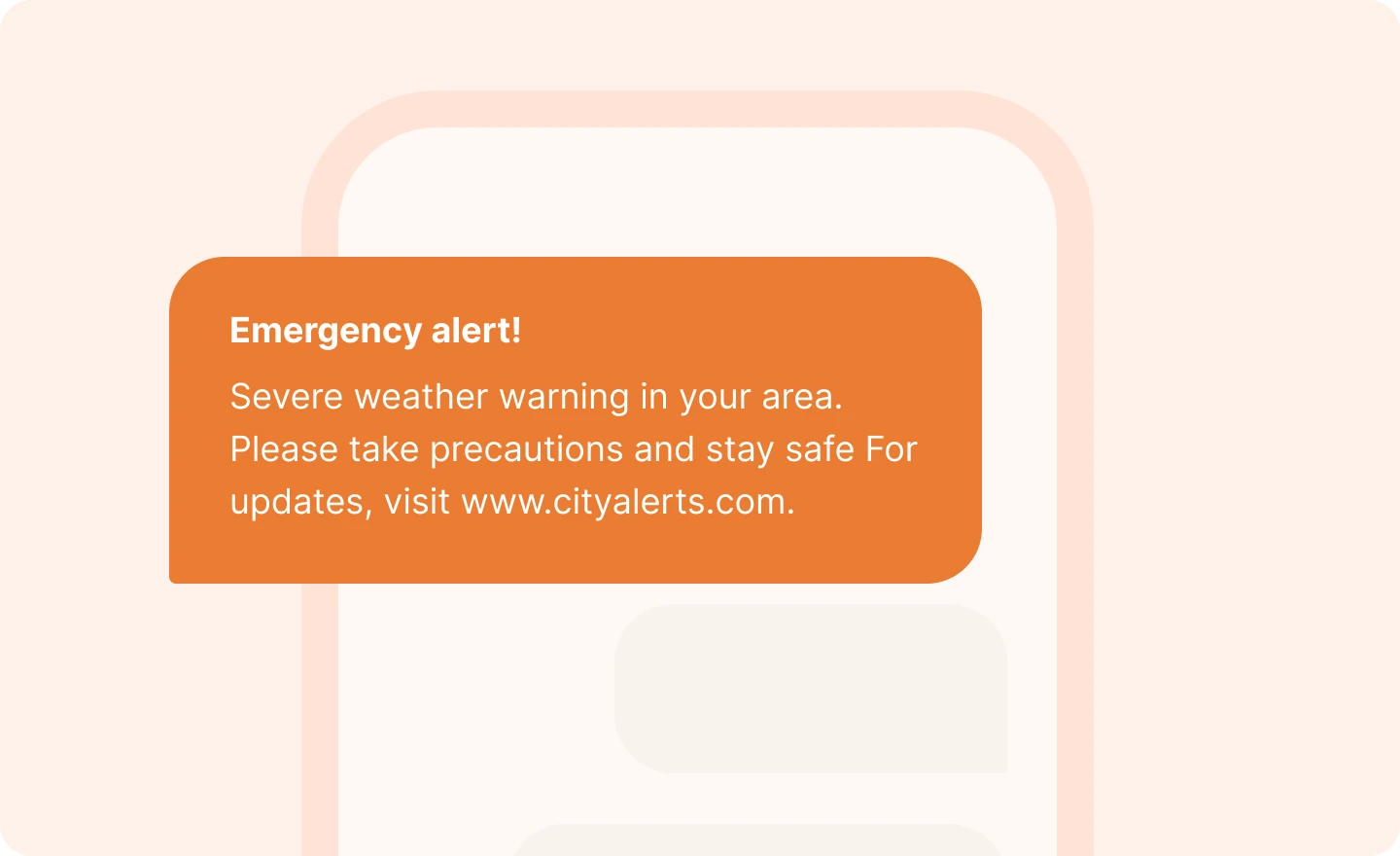
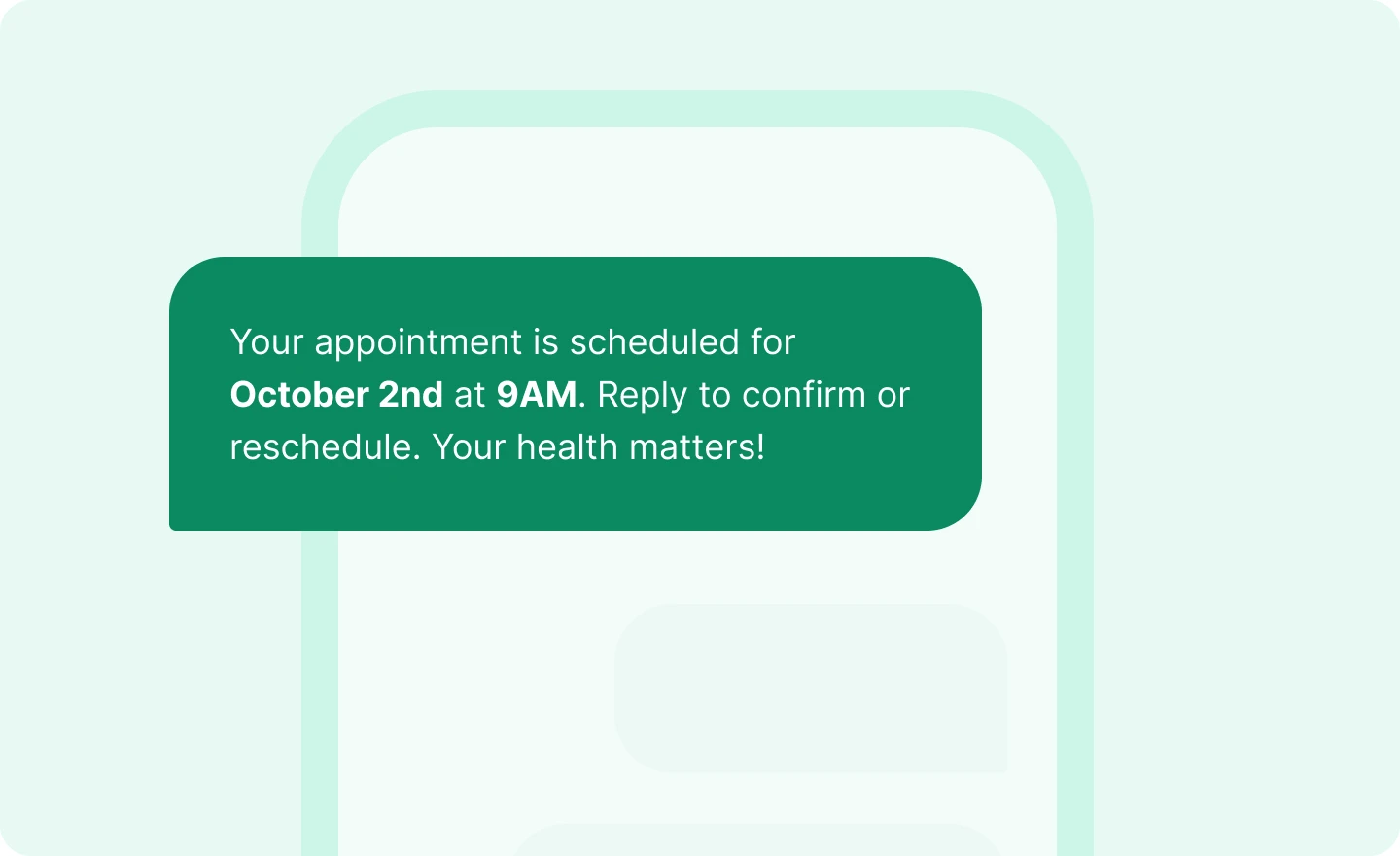
Why choose MailerSend’s bulk SMS API
Super scalability
The advanced bulk SMS API and flexible pricing plans make scaling your SMS sending super simple
Fast delivery
A robust sending infrastructure and high-throughput toll-free numbers handle the fast delivery of thousands of SMS messages.
TCPA-compliant
MailerSend is a fully TCPA-compliant bulk SMS service that follows anti-spam and privacy regulations.
Enhanced security
The latest security and encryption protocols along with account security features ensure your account and data are protected.
Award-winning support
Access 24/7 email and live chat support from a friendly and knowledgeable team of human experts.
One transactional communication platform
Manage transactional SMS and emails on a single platform built by deliverability experts.
Reliable bulk SMS messaging API service
Hobby
Starter
Professional
Enterprise
Frequently Asked Questions
How can I integrate the bulk SMS API into my application?
MailerSend’s bulk SMS API can be easily integrated using our 7 official SDKs and no-code integrations with Zapier, Make, and Airtable.
Can I schedule bulk SMS messages to be sent at a specific time?
Yes, you can schedule bulk SMS messages to be sent at a specific time.
Can I send bulk SMS messages internationally?
MailerSend’s SMS feature is currently available for use in North America. Additional regions are coming soon.
Can I track the delivery and response rates of my SMS messages in real time?
The SMS activity dashboard allows you to monitor the real-time status of each SMS message that you send.
Is there a limit to the number of messages I can send at once?
There is a limit of 60 API requests per minute, however you can include up to 50 recipients per request.
How do I personalize bulk SMS messages for each recipient?
MailerSend supports personalization variables so that you can personalize bulk SMS messages with recipients’ names, account details, order information, and so on. See how you can start sending SMS with MailerSend.
Is it possible to automate the sending of bulk SMS based on specific triggers or events?
Yes, with webhooks and other integrations you can trigger SMS messages based on specific activity and events.
What support is available if I encounter issues with the bulk SMS API?
You can contact our friendly customer support team at any time via email or live chat and they’ll be happy to help.